Java根据坐标和半径求圆
时间: 2024-12-22 14:27:00 浏览: 3
在Java中,你可以创建一个Circle类,它包含属性如x坐标、y坐标以及半径,然后提供计算圆面积和周长的方法。这里是一个简单的示例:
```java
public class Circle {
private double centerX;
private double centerY;
private double radius;
// 构造函数
public Circle(double centerX, double centerY, double radius) {
this.centerX = centerX;
this.centerY = centerY;
this.radius = radius;
}
// 计算圆的面积
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
// 计算圆的周长
public double getCircumference() {
return 2 * Math.PI * radius;
}
// 根据给定的坐标和半径更新实例变量
public void setLocationAndRadius(double newX, double newY, double newRadius) {
centerX = newX;
centerY = newY;
radius = newRadius;
}
// 打印圆的信息
@Override
public String toString() {
return "Circle [centerX=" + centerX + ", centerY=" + centerY + ", radius=" + radius + "]";
}
}
// 使用示例
public static void main(String[] args) {
Circle circle = new Circle(0, 0, 5);
System.out.println("Circle area: " + circle.getArea());
System.out.println("Circle circumference: " + circle.getCircumference());
circle.setLocationAndRadius(3, 4, 3); // 更新坐标和半径
System.out.println(circle);
}
```
在这个例子中,通过Circle对象可以获取并操作任意位置和大小的圆,这就是多态的一个体现,因为`getArea()`和`getCircumference()`方法的行为取决于具体的Circle实例。
阅读全文
相关推荐
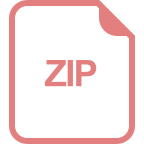















