哈夫曼编码 :设需要编码的字符集为{d1, d2, ..., dn},它们出现的频率为{w1, w2, ..., wn},应用哈夫曼树构造最短的不等长编码方案。使用C语言设计贪心算法求解此哈夫曼编码方案;
时间: 2024-02-18 08:03:16 浏览: 120
以下是使用C语言设计贪心算法求解哈夫曼编码方案的步骤:
1. 定义哈夫曼树节点结构体,包含字符、权值、左右子节点指针等信息。
```c
struct huffman_node {
char ch; // 字符
int weight; // 权值
struct huffman_node *left, *right; // 左右子节点指针
};
```
2. 定义优先队列,用于存储待处理的哈夫曼树节点。
```c
struct priority_queue {
int size; // 队列大小
struct huffman_node **data; // 数据指针数组
};
```
3. 定义哈夫曼编码表,用于存储每个字符对应的编码信息。
```c
struct huffman_table {
char ch; // 字符
char *code; // 编码字符串
};
```
4. 定义比较函数,用于优先队列中节点的比较。
```c
int compare(struct huffman_node *a, struct huffman_node *b) {
return a->weight - b->weight;
}
```
5. 定义创建哈夫曼树的函数。
```c
struct huffman_node* create_huffman_tree(int *weights, int n) {
// 初始化优先队列
struct priority_queue *q = create_priority_queue(n, compare);
for (int i = 0; i < n; i++) {
struct huffman_node *node = (struct huffman_node*)malloc(sizeof(struct huffman_node));
node->ch = i + 'a';
node->weight = weights[i];
node->left = node->right = NULL;
insert(q, node);
}
// 构造哈夫曼树
while (q->size > 1) {
struct huffman_node *node1 = pop(q);
struct huffman_node *node2 = pop(q);
struct huffman_node *parent = (struct huffman_node*)malloc(sizeof(struct huffman_node));
parent->ch = 0;
parent->weight = node1->weight + node2->weight;
parent->left = node1;
parent->right = node2;
insert(q, parent);
}
// 返回根节点
struct huffman_node *root = pop(q);
destroy_priority_queue(q);
return root;
}
```
6. 定义递归函数,生成哈夫曼编码表。
```c
void generate_huffman_table(struct huffman_table *table, struct huffman_node *node, char *code, int depth) {
if (node->left == NULL && node->right == NULL) {
table[node->ch - 'a'].ch = node->ch;
table[node->ch - 'a'].code = (char*)malloc((depth + 1) * sizeof(char));
strcpy(table[node->ch - 'a'].code, code);
} else {
code[depth] = '0';
code[depth + 1] = '\0';
generate_huffman_table(table, node->left, code, depth + 1);
code[depth] = '1';
generate_huffman_table(table, node->right, code, depth + 1);
}
}
```
7. 定义打印哈夫曼编码表的函数。
```c
void print_huffman_table(struct huffman_table *table, int n) {
printf("Character\tCode\n");
for (int i = 0; i < n; i++) {
printf("%c\t\t%s\n", table[i].ch, table[i].code);
}
}
```
8. 最后,调用上述函数生成哈夫曼编码方案。
```c
int main() {
int weights[] = {5, 2, 4, 7, 1, 3};
int n = sizeof(weights) / sizeof(weights[0]);
struct huffman_node *root = create_huffman_tree(weights, n);
struct huffman_table table[26];
for (int i = 0; i < 26; i++) {
table[i].ch = 0;
table[i].code = NULL;
}
char code[100];
code[0] = '\0';
generate_huffman_table(table, root, code, 0);
print_huffman_table(table, 26);
return 0;
}
```
以上代码中,权值数组weights和字符集大小n可以根据具体需要修改。
阅读全文
相关推荐
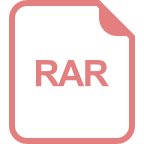

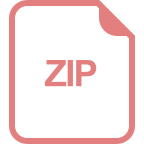
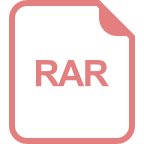
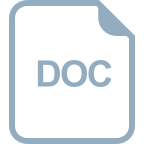
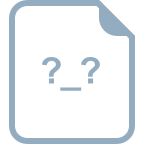
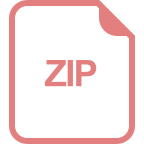
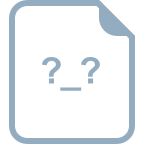
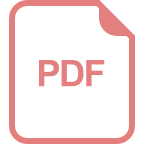
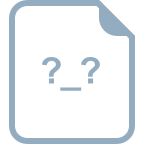
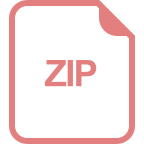
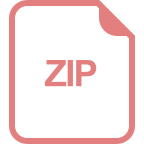
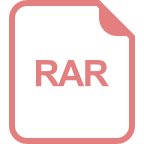
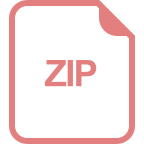
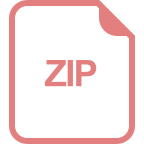