您给的代码触发 MessageBox后再次按任何按键都会触发
时间: 2023-07-15 14:15:08 浏览: 94
这是因为代码中使用了 `MessageBox` 函数的 `MB_SYSTEMMODAL` 参数,它会创建一个系统模态对话框,并且该对话框将一直保持在最前面,直到用户响应为止。
如果您想要在用户响应后才能继续执行代码,可以将 `MessageBox` 的参数改为 `MB_OK` 或者使用其他类型的对话框,比如 `MessageBoxA` 和 `MessageBoxW` 中的 `MB_OKCANCEL`。这样,在用户点击“确定”或“取消”按钮之前,代码将被阻塞。
以下是一个示例代码:
```cpp
#include <Windows.h>
int main()
{
MessageBox(NULL, "Hello World!", "Message Box Example", MB_OK);
return 0;
}
```
在上面的代码中,我将 `MessageBox` 的参数改为了 `MB_OK`,这意味着用户需要点击“确定”按钮才能继续执行代码。
相关问题
四个按键能够改变温湿度阈值大小的代码
在编写这样的代码时,通常会涉及到用户界面设计以及一些基本的数据处理逻辑。假设我们正在创建一个控制温湿度的小型应用程序,可以采用类似以下的Python示例(基于Tkinter库,用于GUI编程):
```python
import tkinter as tk
from tkinter import messagebox
# 定义初始阈值
temperature_threshold = 25
humidity_threshold = 50
def update_threshold(channel):
if channel == 'temperature':
new_value = int(entry_temperature.get())
if new_value > 0:
temperature_threshold = new_value
messagebox.showinfo("更新", f"温度阈值已设置为 {new_value}")
elif channel == 'humidity':
new_value = int(entry_humidity.get())
if new_value > 0:
humidity_threshold = new_value
messagebox.showinfo("更新", f"湿度阈值已设置为 {new_value}")
root = tk.Tk()
tk.Label(root, text="温度阈值").grid(row=0)
entry_temperature = tk.Entry(root)
entry_temperature.grid(row=0, column=1)
button_temperature_increase = tk.Button(root, text="+1", command=lambda: update_threshold('temperature'))
button_temperature_increase.grid(row=0, column=2)
tk.Label(root, text="湿度阈值").grid(row=1)
entry_humidity = tk.Entry(root)
entry_humidity.grid(row=1, column=1)
button_humidity_increase = tk.Button(root, text="+1", command=lambda: update_threshold('humidity'))
button_humidity_increase.grid(row=1, column=2)
root.mainloop()
```
在这个例子中,我们有两个输入框(entry_temperature 和 entry_humidity)让用户输入新的温湿度阈值,以及两个按钮(分别增加温度和湿度阈值)。当用户点击相应按钮时,`update_threshold` 函数会被触发,并根据指定的通道('temperature' 或 'humidity')更新阈值。
如何给WinForm的窗体添加按键监听
要给 WinForm 窗体添加按键监听,你可以使用窗体的 `KeyDown` 或 `KeyPress` 事件。以下是一个示例代码:
```csharp
using System;
using System.Windows.Forms;
namespace KeyListenerExample
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
private void MainForm_KeyDown(object sender, KeyEventArgs e)
{
// 按下任意键时触发
if (e.KeyCode == Keys.Escape)
{
// 如果按下的是 Esc 键,关闭窗体
this.Close();
}
}
private void MainForm_KeyPress(object sender, KeyPressEventArgs e)
{
// 按下字符键时触发
if (e.KeyChar == ' ')
{
// 如果按下的是空格键,显示一个消息框
MessageBox.Show("你按下了空格键!");
}
}
}
static class Program
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
}
}
```
在上面的示例中,我们在 `MainForm` 类中订阅了 `KeyDown` 和 `KeyPress` 事件。在 `MainForm_KeyDown` 方法中,我们检查按下的键是否是 Esc 键,如果是,则关闭窗体。在 `MainForm_KeyPress` 方法中,我们检查按下的键是否是空格键,如果是,则显示一个消息框。
请注意,上述代码是在 Windows 窗体应用程序中使用的示例。你需要创建一个新的 Windows 窗体应用程序项目,并将代码放置在主窗体类中。
阅读全文
相关推荐
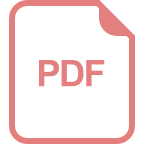
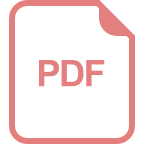
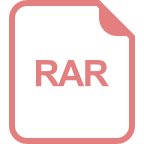
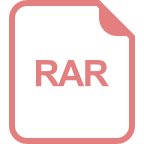
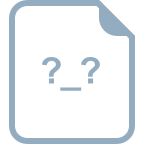
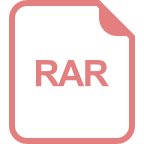
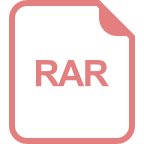
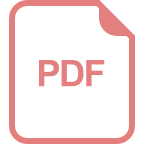
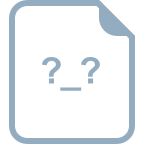
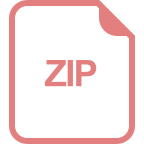
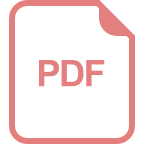
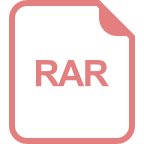
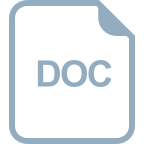
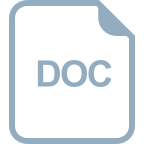
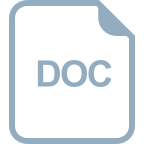

