c++随机输入一个字符串,并逆序输出它
时间: 2024-01-10 13:02:50 浏览: 119
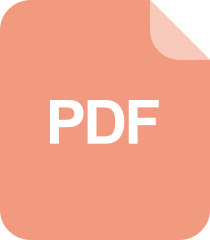
C++输入字符串逆序输出
可以使用`std::getline`函数读取一行字符串,再使用`std::reverse`函数逆序输出。下面是示例代码:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str;
std::cout << "请输入一个字符串:" << std::endl;
std::getline(std::cin, str);
std::reverse(str.begin(), str.end());
std::cout << "逆序输出:" << str << std::endl;
return 0;
}
```
示例输出:
```
请输入一个字符串:
Hello, world!
逆序输出:!dlrow ,olleH
```
阅读全文
相关推荐
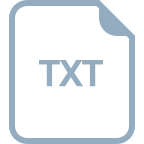
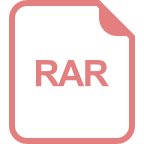
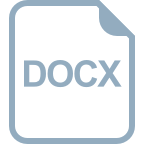
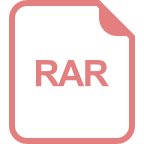
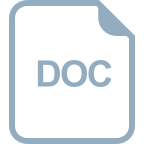
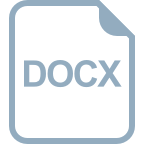
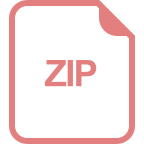
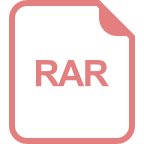
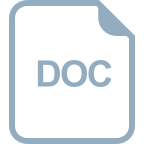
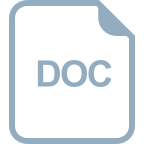
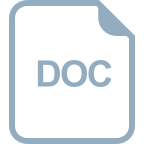
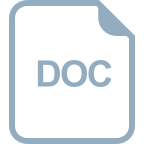
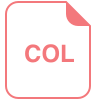
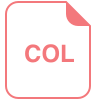