svm图片识别自行拍照,6个分类以上 1.读取数据 2.分割数据集为测试数据集,训练数据集 2.提取特征(降度) 3.在训练集上训练SVM训练模型 4.在测试数据集进行正确率绘制(核函数选择要有两个以上)代码
时间: 2024-10-21 19:09:20 浏览: 11
在使用支持向量机(SVM)进行图像分类的步骤中,特别是在有六个类别以上的任务中,以下是基本流程:
1. **读取数据**:
首先,你需要加载图像数据,通常这涉及到读取文件夹下的图片,并将其转换为可以用于机器学习的格式,例如灰度图、颜色直方图或其他特征向量。
```python
import os
from skimage.io import imread
data_folder = 'path/to/image/folder'
images = [imread(os.path.join(data_folder, img_name)) for img_name in os.listdir(data_folder)]
```
2. **数据预处理**:
- 分割数据集:使用如sklearn的`train_test_split`函数将数据分为训练集和测试集。
- 特征提取:对图片应用特征提取技术,如局部二值模式(LBP)、尺度不变特征变换(SIFT)或使用深度学习模型提取的卷积神经网络(CNN)特征。
```python
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(images, labels, test_size=0.2, random_state=42)
from sklearn.feature_extraction.image import extract_patches_2d
features_train, features_test = extract_patches_2d(X_train, ...), extract_patches_2d(X_test, ...)
```
3. **特征降维**:
使用PCA(主成分分析)等降维算法减少特征维度,以便于SVM更快地运行并降低过拟合风险。
```python
from sklearn.decomposition import PCA
pca = PCA(n_components=0.95) # 或者尝试不同的维度比例
features_train_pca = pca.fit_transform(features_train)
features_test_pca = pca.transform(features_test)
```
4. **训练SVM模型**:
- 实例化SVM模型,可以选择线性核(linear)、多项式核(poly)或径向基函数(RBF)核。
- 训练模型,设置合适的惩罚参数C和内核参数(比如gamma)。
```python
from sklearn.svm import SVC
svm_model = SVC(kernel='rbf', C=1, gamma='scale') # 核函数选择其中之一
svm_model.fit(features_train_pca, y_train)
```
5. **评估与绘制曲线**:
使用交叉验证(如GridSearchCV)优化超参数,然后在测试集上计算准确率。绘制不同核函数下准确率对比图,可以帮助你选择最合适的模型。
```python
from sklearn.model_selection import GridSearchCV
param_grid = {'kernel': ['linear', 'poly', 'rbf'], 'C': [0.1, 1, 10], 'gamma': ['scale', 'auto']}
grid_search = GridSearchCV(SVC(), param_grid, cv=5)
grid_search.fit(features_train_pca, y_train)
best_params = grid_search.best_params_
model_with_best_params = SVC(**best_params)
model_with_best_params.fit(features_train_pca, y_train)
# 计算准确率并绘制曲线
from sklearn.metrics import accuracy_score
y_pred = model_with_best_params.predict(features_test_pca)
accuracy = accuracy_score(y_test, y_pred)
plot_accuracy_curve(best_params['kernel'], accuracy)
```
阅读全文
相关推荐
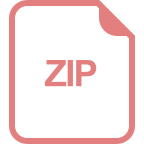
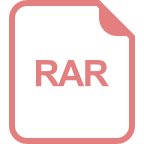
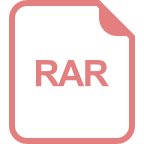















