我想用wapper来书写
时间: 2023-12-19 11:05:52 浏览: 122
好的,使用 Mybatis-Plus 的 QueryWrapper 来书写该 SQL 查询语句的步骤如下:
1. 在 Blog 实体类中添加对应的属性和注解,例如:
```
public class Blog {
private Long id;
private String title;
private String password;
private LocalDateTime createTime;
private String firstPicture;
private Boolean isPublished;
private Boolean isRecommend;
// getter 和 setter 略
}
```
2. 在 BlogMapper 接口中添加对应的方法,例如:
```
public interface BlogMapper extends BaseMapper<Blog> {
List<Blog> selectPublishedAndRecommendedBlogs(Integer limitNum);
}
```
3. 在 BlogMapper.xml 文件中编写对应的 SQL 查询语句,例如:
```
<select id="selectPublishedAndRecommendedBlogs" resultType="com.example.demo.entity.Blog">
select id, title, password, create_time, first_picture
from blog
where is_published = true and is_recommend = true
order by rand()
<if test="limitNum != null">
limit #{limitNum}
</if>
</select>
```
4. 在 BlogMapper 接口的实现类中,调用 selectPublishedAndRecommendedBlogs 方法并传入对应的参数,例如:
```
@Service
public class BlogServiceImpl implements BlogService {
@Autowired
private BlogMapper blogMapper;
@Override
public List<Blog> getPublishedAndRecommendedBlogs(Integer limitNum) {
QueryWrapper<Blog> wrapper = new QueryWrapper<>();
wrapper.eq("is_published", true)
.eq("is_recommend", true)
.orderByAsc("rand()");
if (limitNum != null) {
wrapper.last("limit " + limitNum);
}
return blogMapper.selectList(wrapper);
}
}
```
其中,QueryWrapper 用于构造查询条件,可以通过调用 eq 方法设置相应的筛选条件,例如 eq("is_published", true) 表示筛选 is_published 属性为 true 的博客。orderByAsc("rand()") 表示按照 rand() 函数的结果升序排序,last("limit " + limitNum) 表示添加 limit 子句并设置结果数量。最后,调用 blogMapper.selectList(wrapper) 执行查询并返回结果。
这样就可以使用 Mybatis-Plus 的 QueryWrapper 来进行 SQL 查询语句的编写了。
阅读全文
相关推荐
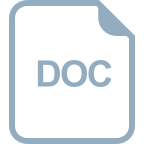
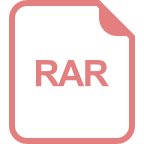

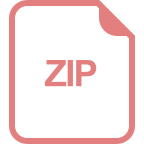
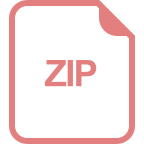
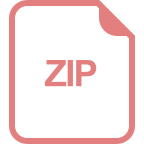
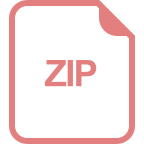
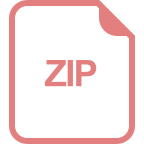
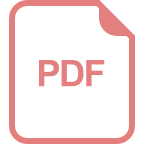
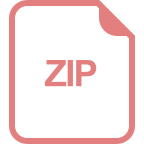
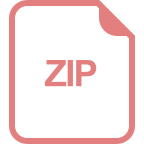
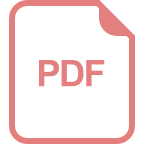







