#include<iostream> #include<fstream> #include<string> #include <algorithm> using namespace std; #define MAXSIZE 10000 #define KEYSIZE 10 #define OK 0 #define ERROR -1 typedef string KeyType; typedef struct { KeyType key; int count; int index; }ElemType; typedef struct { ElemType *R; int length; }SSTable; KeyType key[KEYSIZE] = {"little","prince","sheep","flowers","believe","stars","one","my","he","the"}; int InitSSTable(SSTable &ST) { /*-----------代码开始--------------*/ /*-----------代码结束--------------*/ return OK; } int InsertSSTable(SSTable &ST,KeyType key,int index) { ST.length++; /*-----------代码开始--------------*/ /*-----------代码结束--------------*/ ST.R[ST.length].index = index; return OK; } string SplitWord(string str) { int begin, end; for(begin=0;begin<str.length();begin++) { if(str[begin]>='a' && str[begin]<='z') break; } for(end=str.length()-1;end>=0;end--) { if(str[end]>='a' && str[end]<='z') break; } if(begin<=end) return str.substr(begin,end-begin+1); else return ""; } char op(char c) { if(c>='A' && c<='Z') c = c+32; return c; } int ProcessIn(KeyType *test,int &len,ifstream &in) { int i = 0; string temp; while(!in.eof()) { in>>temp; transform(temp.begin(), temp.end(), temp.begin(), op); test[i] = SplitWord(temp); i++; } len = i; return OK; } int SearchBin(SSTable ST,KeyType key) { /*-----------代码开始--------------*/ /*-----------代码结束--------------*/ return 0; } void Show(SSTable ST) { for(int i=1;i<=KEYSIZE;i++) cout<<ST.R[i].key<<":"<<ST.R[i].count<<endl; } bool CmpKey(ElemType x,ElemType y) { return x.key < y.key; } bool CmpIndex(ElemType x,ElemType y) { return x.index < y.index; } int main() { ifstream in("testData/小王子.txt"); SSTable ST; KeyType test[MAXSIZE]; int len; ProcessIn(test,len,in); InitSSTable(ST); for(int i=0;i<KEYSIZE;i++) InsertSSTable(ST,key[i],i); sort(ST.R+1,ST.R+1+KEYSIZE,CmpKey); //统计关键词列表中单词的词频 /*-----------代码开始--------------*/ /*-----------代码结束--------------*/ sort(ST.R+1,ST.R+1+KEYSIZE,CmpIndex); Show(ST); in.close(); return OK; }
时间: 2023-08-27 17:22:39 浏览: 50
这段代码是实现基于折半查找的词频统计的程序,主要包括以下几个函数:
1. InitSSTable:初始化SSTable,即创建一个空表,用于存储关键词及其出现次数和位置信息;
2. InsertSSTable:向SSTable中插入一个关键词,如果表中已存在该关键词,则将其出现次数加1;
3. SplitWord:将输入的字符串按单词切分,并过滤掉非字母字符;
4. ProcessIn:处理输入文件,将其中的单词切分出来并存储在一个字符串数组中;
5. SearchBin:基于折半查找算法,在SSTable中查找关键词,并返回其在表中的位置;
6. Show:输出SSTable中所有关键词及其出现次数。
程序的基本思路是,先将关键词列表中的关键词插入到SSTable中,然后对输入文件中的单词进行处理,对于每个单词,先使用SplitWord过滤非字母字符,然后使用SearchBin在SSTable中查找该单词是否已存在,如果存在则将其出现次数加1,否则将其插入到SSTable中。最后,对SSTable中的关键词按照字典序排序,并输出每个关键词及其出现次数。
相关问题
#include<iostream> #include<cstdio> using namespace std;是什么意思
#include<iostream> #include<cstdio> using namespace std; 是C++中的预处理指令,用于引入头文件和命名空间。
1. #include<iostream> 是引入iostream头文件,其中包含了输入输出流的定义,例如cout和cin等。
2. #include<cstdio> 是引入cstdio头文件,其中包含了C语言标准输入输出函数的定义,例如printf和scanf等。
3. using namespace std; 是使用std命名空间,std是C++标准库的命名空间,其中包含了很多常用的函数和类。
这些预处理指令的作用是为了在程序中能够使用输入输出流和标准库函数,使得程序更加方便和简洁。
#include <IOSTREAM> #include <FSTREAM>
`#include <iostream>` 和 `#include <fstream>` 是C++中的两个常用的标准库头文件。
- `#include <iostream>` 是用于输入和输出操作的头文件。它定义了 `cout`、`cin` 和 `cerr` 等对象,以及用于标准输入、标准输出和标准错误输出的流对象。通过包含这个头文件,可以在程序中使用输入输出流来进行交互和输出信息。
- `#include <fstream>` 是用于文件操作的头文件。它定义了 `ifstream`、`ofstream` 和 `fstream` 等类,可以用于读取和写入文件。通过包含这个头文件,可以在程序中使用文件流对象来打开、读取和写入文件。
需要注意的是,头文件名字中的字母是大小写敏感的,应该按照正确的大小写来包含这两个头文件,即 `<iostream>` 和 `<fstream>`。此外,这两个头文件都不需要添加额外的扩展名,例如 `.h` 或 `.hpp`。
相关推荐
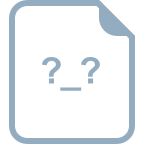













