include <iostream>#include <cstdlib> #include <ctime>
时间: 2024-04-14 15:25:09 浏览: 223
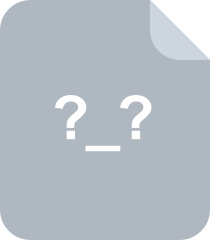
#include <iostream>
这是C++代码,用于包含头文件iostream、cstdlib和ctime。这些头文件提供了一些常用的函数和类,例如输入输出流、随机数生成和时间函数等。下面是一个简单的示例代码,演示了如何使用这些头文件中的函数:
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
// 生成随机数种子
std::srand(std::time(0));
// 生成一个随机数
int randomNumber = std::rand();
// 输出随机数
std::cout << "Random number: " << randomNumber << std::endl;
return 0;
}
```
这段代码首先使用`std::time(0)`函数获取当前时间作为随机数种子,然后使用`std::srand()`函数将种子传递给随机数生成器。接下来,使用`std::rand()`函数生成一个随机数,并使用`std::cout`输出到控制台。
阅读全文
相关推荐
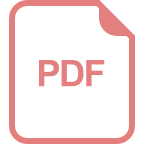
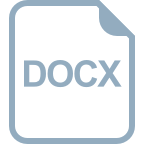
















