#include <iostream>#include <cstdlib>#include <ctime>#include <cstring>using namespace std;const int MAX_WRONG = 6; //最大错误次数string WORDS[] = {"HELLO", "WORLD", "COMPUTER", "PROGRAMMING", "LANGUAGE"}; //单词库int main(){ srand(time(0)); //用当前时间作为随机数种子 char play; //玩家选择是否继续游戏 do { string word = WORDS[rand() % 5]; //从单词库中随机选择一个单词 int wrong = 0; //错误次数 string soFar(word.size(), '_'); //初始时,所有字母都用"_"代替 string used = ""; //已经猜过的字母 cout << "Welcome to Hangman. Good luck!\n"; //循环直到猜出单词或者错误次数达到最大值 while (wrong < MAX_WRONG && soFar != word) { cout << "\n\nYou have " << (MAX_WRONG - wrong) << " incorrect guesses left.\n"; cout << "\nYou've used the following letters:\n" << used << endl; cout << "\nSo far, the word is:\n" << soFar << endl; char guess; //玩家猜测的字母 cout << "\n\nEnter your guess: "; cin >> guess; guess = toupper(guess); //将小写字母转换成大写字母 while (used.find(guess) != string::npos) { cout << "\nYou've already guessed " << guess << endl; cout << "Enter your guess: "; cin >> guess; guess = toupper(guess); } used += guess; if (word.find(guess) != string::npos) { cout << "That's right! " << guess << " is in the word.\n"; for (int i = 0; i < word.size(); ++i) { if (word[i] == guess) { soFar[i] = guess; } } } else { cout << "Sorry, " << guess << " isn't in the word.\n"; ++wrong; } } if (wrong == MAX_WRONG) { cout << "\nYou've been hanged!"; } else { cout << "\nYou guessed it!"; } cout << "\nThe word was " << word << endl; cout << "\nDo you want to play again? (y/n): "; cin >> play; play = toupper(play); } while (play == 'Y'); return 0;}优化这段代码
时间: 2023-12-10 10:41:52 浏览: 81
以下是优化后的代码:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <string>
#include <algorithm>
#include <cctype>
using namespace std;
const int MAX_WRONG = 6;
const string WORDS[] = {"HELLO", "WORLD", "COMPUTER", "PROGRAMMING", "LANGUAGE"};
int main() {
srand(time(nullptr)); // 使用nullptr代替0作为空指针常量
char play = 'y';
while (tolower(play) == 'y') { // 使用tolower将玩家输入转换成小写字母
string word = WORDS[rand() % 5];
int wrong = 0;
string soFar(word.size(), '_');
string used;
cout << "Welcome to Hangman. Good luck!\n\n";
while (wrong < MAX_WRONG && soFar != word) {
cout << "You have " << MAX_WRONG - wrong << " incorrect guesses left.\n";
cout << "You've used the following letters:\n" << used << endl;
cout << "So far, the word is:\n" << soFar << endl;
char guess;
cout << "\nEnter your guess: ";
cin >> guess;
guess = toupper(guess);
if (used.find(guess) != string::npos) { // 使用find函数代替while循环
cout << "You've already guessed " << guess << endl;
continue;
}
used += guess;
if (word.find(guess) != string::npos) {
cout << "That's right! " << guess << " is in the word.\n";
for (int i = 0; i < word.size(); ++i) {
if (word[i] == guess) {
soFar[i] = guess;
}
}
} else {
cout << "Sorry, " << guess << " isn't in the word.\n";
++wrong;
}
}
if (wrong == MAX_WRONG) {
cout << "\nYou've been hanged!";
} else {
cout << "\nYou guessed it!";
}
cout << "\nThe word was " << word << endl;
cout << "Do you want to play again? (y/n): ";
cin >> play;
}
return 0;
}
```
主要的改进点如下:
1. 使用nullptr代替0作为空指针常量,使用tolower将玩家输入转换成小写字母。
2. 使用find函数代替while循环,优化猜测重复字母的判断逻辑。
3. 化繁为简,去掉一些不必要的输出。
阅读全文
相关推荐
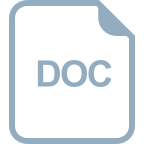
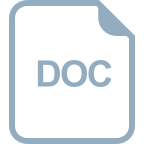
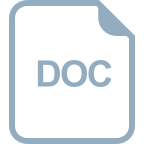






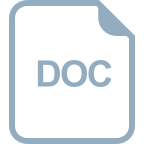
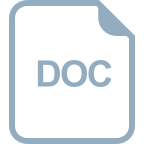
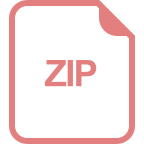