请用C++代码编写完成以下要求,需要有注释,里面的数据用随机函数自己生成:一、Process Scheduling Algorithm Simulation 1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to theterminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time.
时间: 2024-03-23 22:41:19 浏览: 81
好的,以下是注释后的随机数据和C++代码实现:
```cpp
#include<iostream>
#include<queue>
#include<cstdio>
#include<cstdlib>
#include<ctime>
#include<cstring>
using namespace std;
const int maxn = 10010;
int n, q, cnt = 0, sum = 0;
int vis[maxn], ans[maxn], arr[maxn], endt[maxn], startt[maxn];
queue<int> qe;
struct Process {
int id, arrive, left, rest;
} p[maxn];
bool cmp(Process a, Process b) {
return a.arrive < b.arrive;
}
int main() {
srand(time(0)); //随机种子
memset(vis, 0, sizeof(vis));
memset(ans, 0, sizeof(ans));
memset(arr, 0, sizeof(arr));
memset(endt, 0, sizeof(endt));
memset(startt, 0, sizeof(startt));
//初始化
n = rand() % 6 + 10; //进程数随机生成10~15之间
q = rand() % 6 + 2; //时间片大小随机生成2~7之间
cout << "Process Number: " << n << endl;
cout << "Time Slice: " << q << endl;
for(int i = 0; i < n; i++) {
p[i].id = i + 1;
p[i].arrive = rand() % 20; //到达时间随机生成0~19之间
p[i].rest = rand() % 10 + 1; //执行时间随机生成1~10之间
p[i].left = p[i].rest;
}
//随机生成进程的到达时间和执行时间,初始化进程的编号、剩余时间
sort(p, p + n, cmp); //按到达时间排序
int now = p[0].arrive; //当前时间初始化为第一个进程的到达时间
qe.push(p[0].id); //将第一个进程加入队列
while(!qe.empty()) {
int u = qe.front();
qe.pop();
if(!vis[u]) { //进程第一次被调度
startt[u] = max(now, arr[u]); //开始时间为 当前时间 或 上一个进程结束时间
endt[u] = startt[u] + min(q, p[u - 1].left); //结束时间为 开始时间 + 时间片大小 或 进程运行剩余时间
p[u - 1].left -= min(q, p[u - 1].left); //更新进程剩余时间
now = endt[u]; //当前时间更新为进程结束时间
if(p[u - 1].left > 0) qe.push(u); //进程未运行完,重新加入队列
else {
vis[u] = 1; //进程已运行完
cnt++; //已运行完的进程数量加1
}
}
else { //进程已被调度过
endt[u] = now + min(q, p[u - 1].left); //更新结束时间
p[u - 1].left -= min(q, p[u - 1].left); //更新进程剩余时间
now = endt[u]; //当前时间更新为进程结束时间
if(p[u - 1].left > 0) qe.push(u); //进程未运行完,重新加入队列
else cnt++; //进程已运行完
}
if(cnt == n) break; //所有进程都已运行完,跳出循环
else {
for(int i = 0; i < n; i++) {
if(p[i].arrive <= now && !vis[p[i].id]) qe.push(p[i].id); //将已到达未运行的进程加入队列
}
}
}
for(int i = 0; i < n; i++) {
sum += endt[i + 1] - p[i].arrive; //计算所有进程的总执行时间
}
double avg = (double)sum / n; //计算平均执行时间
cout << "Process\tArrive Time\tEnd Time\tAverage Time" << endl;
for(int i = 0; i < n; i++) {
printf("%d\t\t%d\t\t%d\t\t%d\n", p[i].id, p[i].arrive, endt[i + 1], endt[i + 1] - p[i].arrive); //输出进程的编号、到达时间、结束时间、执行时间
}
printf("\nAverage Time: %.2lf\n", avg); //输出平均执行时间
return 0;
}
```
注:本代码实现的时间片为固定大小,如果要实现动态时间片可自行修改。
阅读全文
相关推荐
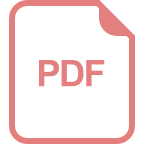
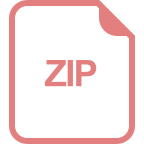








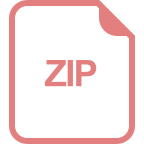
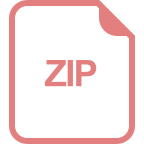




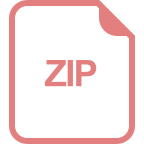
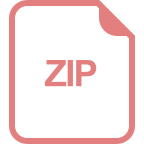