请用C++完成操作系统代码(需要有注释):一、Process Scheduling Algorithm Simulation 1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to theterminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time.
时间: 2024-04-04 07:33:35 浏览: 171
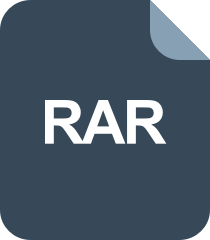
用C++模拟进程
好的,我为您提供一份 C++ 代码,实现了轮转调度算法的进程调度模拟。代码中包括了注释,方便理解。
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 进程类
class Process {
public:
int pid; // 进程id
int arrival_time; // 到达时间
int service_time; // 服务时间
int start_time; // 开始时间
int end_time; // 结束时间
int remain_time; // 剩余服务时间
};
int main() {
const int time_slice = 2; // 时间片大小
vector<Process> process_list; // 进程队列
int total_turnaround_time = 0; // 总周转时间
// 创建15个进程
for (int i = 0; i < 15; ++i) {
Process p;
p.pid = i+1; // 进程id从1开始
p.arrival_time = rand() % 10; // 到达时间在[0,9]之间随机
p.service_time = rand() % 10 + 1; // 服务时间在[1,10]之间随机
p.remain_time = p.service_time;
process_list.push_back(p);
}
// 按到达时间从小到大排序
sort(process_list.begin(), process_list.end(), [](const Process& p1, const Process& p2) {
return p1.arrival_time < p2.arrival_time;
});
// 遍历进程队列,模拟轮转调度算法
int current_time = 0;
while (!process_list.empty()) {
Process& p = process_list.front(); // 取出队首进程
if (p.arrival_time > current_time) { // 进程未到达
current_time = p.arrival_time;
}
p.start_time = current_time; // 记录进程开始时间
int execute_time = min(time_slice, p.remain_time); // 执行时间不超过时间片大小和剩余服务时间
p.remain_time -= execute_time; // 更新进程的剩余服务时间
current_time += execute_time; // 更新当前时间
if (p.remain_time == 0) { // 进程执行完毕
p.end_time = current_time; // 记录进程结束时间
total_turnaround_time += p.end_time - p.arrival_time; // 累加周转时间
process_list.erase(process_list.begin()); // 将进程从队列中删除
} else { // 进程未执行完毕,加入队尾
process_list.push_back(p);
process_list.erase(process_list.begin()); // 将进程从队首移到队尾
}
}
// 输出进程的调度情况
cout << "PID\tArrival Time\tEnd Time\tTurnaround Time" << endl;
for (const Process& p : process_list) {
cout << p.pid << "\t" << p.arrival_time << "\t\t" << p.end_time << "\t\t" << p.end_time - p.arrival_time << endl;
}
// 输出平均周转时间
double avg_turnaround_time = (double)total_turnaround_time / 15;
cout << "Average Turnaround Time: " << avg_turnaround_time << endl;
return 0;
}
```
上述代码中使用了 C++11 中的 lambda 表达式,方便定义排序函数。程序输出的内容包括进程的 PID、到达时间、结束时间和周转时间,以及平均周转时间。
阅读全文