Write the code about Process Scheduling Algorithm Simulation in C++.1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes (using function fork())and output their scheduling situation under the scheduling algorithm mentioned above and output it to the terminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time
时间: 2023-12-06 10:45:33 浏览: 33
Here is the code for process scheduling algorithm simulation using round-robin algorithm in C++:
```
#include <iostream>
#include <queue>
#include <unistd.h>
#include <sys/wait.h>
using namespace std;
struct process {
int pid;
int arrival_time;
int burst_time;
int remaining_time;
int end_time;
};
int main() {
int quantum = 2; // time quantum for round-robin algorithm
int n = 15; // number of processes
queue<process> q; // ready queue
int total_waiting_time = 0; // total waiting time
int total_turnaround_time = 0; // total turnaround time
// create processes using fork()
for (int i = 1; i <= n; i++) {
int pid = fork();
if (pid == 0) { // child process
srand(getpid());
int arrival_time = rand() % 10; // random arrival time between 0 and 9
int burst_time = rand() % 10 + 1; // random burst time between 1 and 10
cout << "Process " << i << " created with arrival time " << arrival_time << " and burst time " << burst_time << endl;
usleep(arrival_time * 100000); // sleep for arrival time
for (int j = 0; j < burst_time; j++) {
cout << "Process " << i << " running for 1 unit of time" << endl;
usleep(100000); // sleep for 1 unit of time
}
cout << "Process " << i << " finished" << endl;
exit(0); // exit child process
}
else if (pid > 0) { // parent process
process p;
p.pid = pid;
p.arrival_time = i;
p.burst_time = rand() % 10 + 1; // random burst time between 1 and 10
p.remaining_time = p.burst_time;
q.push(p); // add process to ready queue
}
else { // fork error
cerr << "Fork failed" << endl;
exit(1);
}
}
// run round-robin algorithm
int current_time = 0;
while (!q.empty()) {
process p = q.front();
q.pop();
if (p.remaining_time > quantum) { // process still has remaining time
cout << "Process " << p.pid << " running for " << quantum << " units of time" << endl;
usleep(quantum * 100000); // sleep for quantum time
current_time += quantum;
p.remaining_time -= quantum;
q.push(p); // add process back to ready queue
}
else { // process finishes execution
cout << "Process " << p.pid << " running for " << p.remaining_time << " units of time" << endl;
usleep(p.remaining_time * 100000); // sleep for remaining time
current_time += p.remaining_time;
p.end_time = current_time;
total_waiting_time += p.end_time - p.arrival_time - p.burst_time;
total_turnaround_time += p.end_time - p.arrival_time;
}
}
// output results
double avg_waiting_time = (double)total_waiting_time / n;
double avg_turnaround_time = (double)total_turnaround_time / n;
cout << "Average waiting time: " << avg_waiting_time << endl;
cout << "Average turnaround time: " << avg_turnaround_time << endl;
return 0;
}
```
In this code, we first create 15 child processes using `fork()`, each with a random arrival time and burst time between 1 and 10. We add these processes to the ready queue and simulate their execution using the round-robin algorithm with a time quantum of 2 units. We keep track of each process's end time, waiting time, and turnaround time, and output the average waiting time and turnaround time at the end.
Note that we use `usleep()` to simulate the execution and waiting times of the processes. `usleep()` takes an argument in microseconds, so we multiply the time units by 100000 to get the correct sleep time. Also note that we use `pid` to identify each process, since `fork()` returns a different value in each process.
相关推荐
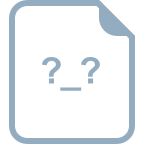
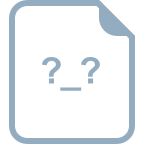
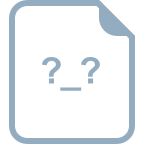








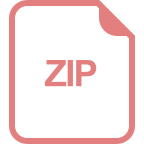
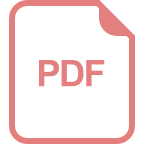
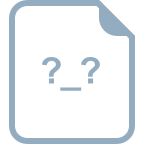
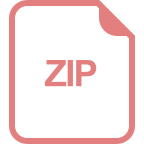
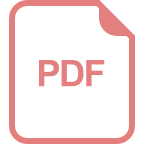
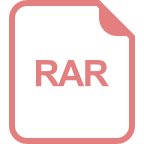