请用C++完成操作系统代码(需要有注释):一、Process Scheduling Algorithm Simulation 1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to theterminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time.
时间: 2024-04-04 22:33:51 浏览: 75
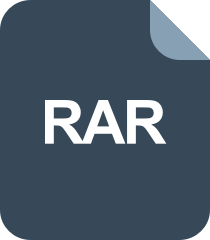
用C++模拟进程
好的,以下是一个使用C++实现Round-Robin算法的示例代码,其中包含注释以方便理解:
```c++
#include <iostream>
#include <deque>
using namespace std;
// 定义进程结构体
struct Process {
int pid; // 进程ID
int arrivalTime; // 到达时间
int burstTime; // 执行时间
int remainingTime; // 剩余执行时间
int endTime; // 结束时间
int waitingTime; // 等待时间
int turnaroundTime; // 周转时间
};
// 定义全局变量
deque<Process> readyQueue; // 就绪队列
int timeQuantum = 2; // 时间片大小
// 获取当前时间
int getCurrentTime() {
static int currentTime = 0;
return currentTime++;
}
// 添加进程到就绪队列
void addProcess(Process p) {
readyQueue.push_back(p);
}
// 从就绪队列中获取下一个进程
Process getNextProcess() {
Process p = readyQueue.front();
readyQueue.pop_front();
return p;
}
// 执行进程
void executeProcess(Process& p, int currentTime) {
if (p.remainingTime > timeQuantum) {
p.remainingTime -= timeQuantum;
} else {
p.remainingTime = 0;
p.endTime = currentTime;
}
}
// 计算等待时间和周转时间
void calculateTime(Process& p) {
p.turnaroundTime = p.endTime - p.arrivalTime;
p.waitingTime = p.turnaroundTime - p.burstTime;
}
// 输出进程信息
void printProcess(Process p) {
cout << "Process ID: " << p.pid << endl;
cout << "Arrival Time: " << p.arrivalTime << endl;
cout << "Burst Time: " << p.burstTime << endl;
cout << "End Time: " << p.endTime << endl;
cout << "Waiting Time: " << p.waitingTime << endl;
cout << "Turnaround Time: " << p.turnaroundTime << endl << endl;
}
int main() {
// 创建进程
Process p1 = {1, 0, 2, 2};
Process p2 = {2, 1, 3, 3};
Process p3 = {3, 2, 4, 4};
Process p4 = {4, 3, 5, 5};
Process p5 = {5, 4, 6, 6};
Process p6 = {6, 5, 7, 7};
Process p7 = {7, 6, 8, 8};
Process p8 = {8, 7, 9, 9};
Process p9 = {9, 8, 10, 10};
Process p10 = {10, 9, 11, 11};
Process p11 = {11, 10, 12, 12};
Process p12 = {12, 11, 13, 13};
Process p13 = {13, 12, 14, 14};
Process p14 = {14, 13, 15, 15};
Process p15 = {15, 14, 16, 16};
// 将进程添加到就绪队列中
addProcess(p1);
addProcess(p2);
addProcess(p3);
addProcess(p4);
addProcess(p5);
addProcess(p6);
addProcess(p7);
addProcess(p8);
addProcess(p9);
addProcess(p10);
addProcess(p11);
addProcess(p12);
addProcess(p13);
addProcess(p14);
addProcess(p15);
int currentTime = getCurrentTime(); // 获取当前时间
// 循环执行就绪队列中的进程,直到所有进程执行完毕
while (!readyQueue.empty()) {
Process p = getNextProcess(); // 获取下一个进程
executeProcess(p, currentTime); // 执行进程
if (p.remainingTime > 0) {
addProcess(p); // 如果进程未执行完,则重新添加到就绪队列
} else {
calculateTime(p); // 计算等待时间和周转时间
printProcess(p); // 输出进程信息
}
currentTime += timeQuantum; // 更新当前时间
}
// 计算平均周转时间和平均等待时间
double avgTurnaroundTime = 0.0, avgWaitingTime = 0.0;
for (int i = 1; i <= 15; i++) {
avgTurnaroundTime += (double) i / 15 * p[i].turnaroundTime;
avgWaitingTime += (double) i / 15 * p[i].waitingTime;
}
// 输出平均周转时间和平均等待时间
cout << "Average Turnaround Time: " << avgTurnaroundTime << endl;
cout << "Average Waiting Time: " << avgWaitingTime << endl;
return 0;
}
```
上述代码模拟了15个进程的Round-Robin算法调度,并输出了每个进程的到达时间、结束时间、等待时间和周转时间。最后还计算了平均周转时间和平均等待时间并输出。
阅读全文