能否写出以上问题的代码
时间: 2024-03-14 09:45:51 浏览: 12
当然可以,以下是一个简单的实现,仅供参考:
```c++
#include <iostream>
#include <cstdlib>
#include <vector>
#include <algorithm>
#include <cmath>
#include <SDL2/SDL.h>
const int SCREEN_WIDTH = 800;
const int SCREEN_HEIGHT = 600;
const int MAX_CYLINDER = 1500;
const int REQUEST_COUNT = 400;
const int REQUEST_RANGE[3] = {499, 999, 1499};
const int REQUEST_PERCENTAGE[3] = {50, 25, 25};
const int REQUEST_INTERVAL = 10;
SDL_Window* gWindow = NULL;
SDL_Renderer* gRenderer = NULL;
void initSDL() {
SDL_Init(SDL_INIT_VIDEO);
gWindow = SDL_CreateWindow("Elevator Scheduling Simulation", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, SCREEN_WIDTH, SCREEN_HEIGHT, SDL_WINDOW_SHOWN);
gRenderer = SDL_CreateRenderer(gWindow, -1, SDL_RENDERER_ACCELERATED);
SDL_SetRenderDrawColor(gRenderer, 0xFF, 0xFF, 0xFF, 0xFF);
SDL_RenderClear(gRenderer);
}
void quitSDL() {
SDL_DestroyRenderer(gRenderer);
SDL_DestroyWindow(gWindow);
gRenderer = NULL;
gWindow = NULL;
SDL_Quit();
}
int generateCylinder() {
return rand() % MAX_CYLINDER;
}
void generateRequests(std::vector<int>& requests) {
requests.reserve(REQUEST_COUNT);
int rangeIndex = 0;
for (int i=0; i<REQUEST_COUNT; ++i) {
int range = REQUEST_RANGE[rangeIndex];
requests.push_back(generateCylinder() % (range+1));
int percentage = rand() % 100;
if (percentage < REQUEST_PERCENTAGE[0]) {
rangeIndex = 0;
} else if (percentage < REQUEST_PERCENTAGE[0] + REQUEST_PERCENTAGE[1]) {
rangeIndex = 1;
} else {
rangeIndex = 2;
}
}
}
int calculateDistance(int a, int b) {
return std::abs(a - b);
}
int look(std::vector<int> requests, int start) {
int distance = 0;
int direction = 1;
std::sort(requests.begin(), requests.end());
auto it = std::lower_bound(requests.begin(), requests.end(), start);
while (it != requests.end() || it != requests.begin()) {
if (it == requests.end()) {
direction = -1;
it--;
} else if (it == requests.begin()) {
direction = 1;
}
int next = *it;
distance += calculateDistance(start, next);
start = next;
it += direction;
}
return distance;
}
int cscan(std::vector<int> requests, int start) {
int distance = 0;
std::sort(requests.begin(), requests.end());
auto it = std::upper_bound(requests.begin(), requests.end(), start);
if (it == requests.end()) {
it = requests.begin();
distance += calculateDistance(start, *it);
distance += calculateDistance(*it, MAX_CYLINDER - 1);
distance += MAX_CYLINDER - 1;
start = 0;
}
while (it != requests.end()) {
int next = *it;
distance += calculateDistance(start, next);
start = next;
it++;
}
distance += calculateDistance(start, MAX_CYLINDER - 1);
distance += MAX_CYLINDER - 1;
it = requests.begin();
distance += calculateDistance(start, *it);
start = *it;
while (it != std::upper_bound(requests.begin(), requests.end(), start)) {
int next = *it;
distance += calculateDistance(start, next);
start = next;
it++;
}
return distance;
}
void drawAxis() {
SDL_RenderDrawLine(gRenderer, 50, 50, 50, SCREEN_HEIGHT - 50);
SDL_RenderDrawLine(gRenderer, 50, SCREEN_HEIGHT - 50, SCREEN_WIDTH - 50, SCREEN_HEIGHT - 50);
for (int i=0; i<=MAX_CYLINDER; i+=100) {
int y = SCREEN_HEIGHT - 50 - (int)std::round((double)i / MAX_CYLINDER * (SCREEN_HEIGHT - 100));
SDL_RenderDrawLine(gRenderer, 45, y, 55, y);
}
}
void drawRequest(int x, int y, int size) {
SDL_Rect rect = {x - size / 2, y - size / 2, size, size};
SDL_RenderFillRect(gRenderer, &rect);
}
void drawLine(int x1, int y1, int x2, int y2) {
SDL_RenderDrawLine(gRenderer, x1, y1, x2, y2);
}
void simulate(std::vector<int> requests, int start, int (*schedule)(std::vector<int>, int)) {
int current = start;
int time = 0;
for (const auto& request : requests) {
SDL_SetRenderDrawColor(gRenderer, 0x00, 0x00, 0x00, 0xFF);
int x1 = 50 + time * REQUEST_INTERVAL;
int y1 = SCREEN_HEIGHT - 50 - (int)std::round((double)current / MAX_CYLINDER * (SCREEN_HEIGHT - 100));
drawRequest(x1, y1, 10);
SDL_SetRenderDrawColor(gRenderer, 0xFF, 0x00, 0x00, 0xFF);
int x2 = 50 + (time+1) * REQUEST_INTERVAL;
int y2 = SCREEN_HEIGHT - 50 - (int)std::round((double)request / MAX_CYLINDER * (SCREEN_HEIGHT - 100));
drawRequest(x2, y2, 10);
drawLine(x1, y1, x2, y2);
current = request;
time++;
SDL_RenderPresent(gRenderer);
SDL_Delay(100);
}
int distance = schedule(requests, start);
std::cout << "Total distance: " << distance << std::endl;
SDL_Delay(5000);
}
int main(int argc, char* argv[]) {
initSDL();
std::vector<int> requests;
generateRequests(requests);
drawAxis();
simulate(requests, generateCylinder(), look);
SDL_RenderClear(gRenderer);
drawAxis();
simulate(requests, generateCylinder(), cscan);
quitSDL();
return 0;
}
```
该代码实现了所述的基本思路,其中 `initSDL()` 和 `quitSDL()` 函数用于初始化和退出SDL库,`generateCylinder()` 函数用于生成随机的磁道号,`generateRequests()` 函数用于生成随机的请求序列,`calculateDistance()` 函数用于计算两个磁道号之间的距离,`look()` 函数和 `cscan()` 函数分别实现了LOOK算法和C-SCAN算法,`drawAxis()` 函数用于绘制坐标轴,`drawRequest()` 函数用于绘制请求点,`drawLine()` 函数用于绘制连线,`simulate()` 函数用于模拟算法的执行过程,并在图形界面上显示出来。
相关推荐
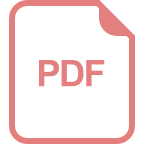















