Write the code about Process Scheduling Algorithm Simulation in C++.The requirements are as follows:1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes (use function fork()) and output their scheduling situation under the scheduling algorithm mentioned above and output it to the terminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time
时间: 2023-12-10 07:40:28 浏览: 120
Here is an example code for Process Scheduling Algorithm Simulation using Round-Robin in C++:
```
#include <iostream>
#include <queue>
#include <ctime>
#include <unistd.h>
using namespace std;
struct Process {
int pid; // Process ID
int arrivalTime; // Arrival Time
int burstTime; // Burst Time
int remainingTime; // Remaining Time
};
int main() {
const int TIME_QUANTUM = 2;
const int NUM_PROCESSES = 15;
queue<Process> readyQueue;
Process processList[NUM_PROCESSES];
int totalExecutionTime = 0, totalWaitTime = 0, currentTime = 0;
// Generate random processes
srand(time(0));
for (int i = 0; i < NUM_PROCESSES; i++) {
processList[i].pid = i;
processList[i].arrivalTime = rand() % 10;
processList[i].burstTime = rand() % 10 + 1;
processList[i].remainingTime = processList[i].burstTime;
totalExecutionTime += processList[i].burstTime;
}
// Sort process list by arrival time
for (int i = 0; i < NUM_PROCESSES - 1; i++) {
for (int j = i + 1; j < NUM_PROCESSES; j++) {
if (processList[i].arrivalTime > processList[j].arrivalTime) {
swap(processList[i], processList[j]);
}
}
}
// Run round-robin scheduling algorithm
int currentProcessIndex = 0;
while (currentTime < totalExecutionTime) {
// Check for newly arrived processes and add them to the ready queue
while (currentProcessIndex < NUM_PROCESSES && processList[currentProcessIndex].arrivalTime <= currentTime) {
readyQueue.push(processList[currentProcessIndex]);
currentProcessIndex++;
}
if (!readyQueue.empty()) {
// Get the next process from the ready queue
Process currentProcess = readyQueue.front();
readyQueue.pop();
// Execute the process for the time quantum or until it finishes
int executionTime = min(TIME_QUANTUM, currentProcess.remainingTime);
currentProcess.remainingTime -= executionTime;
currentTime += executionTime;
// Check if the process has finished
if (currentProcess.remainingTime == 0) {
cout << "Process " << currentProcess.pid << " finished at time " << currentTime << endl;
totalWaitTime += currentTime - currentProcess.arrivalTime - currentProcess.burstTime;
} else {
// Put the process back in the ready queue
readyQueue.push(currentProcess);
}
} else {
// No processes in the ready queue, advance time
currentTime++;
}
}
// Calculate average execution time and output results
double averageExecutionTime = (double)(totalExecutionTime) / NUM_PROCESSES;
double averageWaitTime = (double)(totalWaitTime) / NUM_PROCESSES;
cout << "Average Execution Time: " << averageExecutionTime << endl;
cout << "Average Wait Time: " << averageWaitTime << endl;
return 0;
}
```
This code generates 15 random processes with arrival time and burst time. It then sorts the process list by arrival time and runs the round-robin scheduling algorithm with a time quantum of 2. The program outputs the process ID and finish time for each process and calculates the average execution time and average wait time.
阅读全文
相关推荐
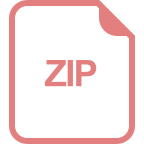
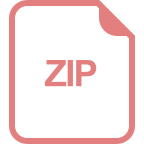
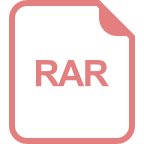








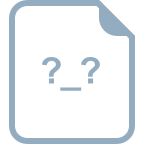
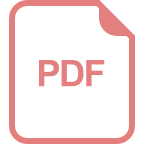
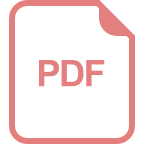
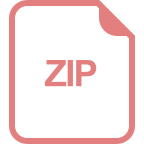
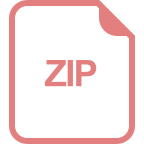