Write the code in C++ with 3 requirements:1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to the terminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time
时间: 2024-01-25 13:02:21 浏览: 109
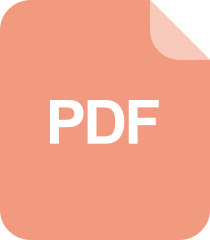
algorithms in C++

Here's the code that simulates the round-robin algorithm for process scheduling in C++:
```
#include <iostream>
#include <queue>
using namespace std;
struct Process {
int pid;
int arrivalTime;
int burstTime;
int remainingTime;
int endTime;
int turnaroundTime;
int waitingTime;
};
void roundRobinScheduling(Process processes[], int n, int quantum) {
queue<Process> readyQueue;
int currentTime = 0;
int completedProcesses = 0;
int totalWaitingTime = 0;
int totalTurnaroundTime = 0;
// Add all processes to the ready queue
for (int i = 0; i < n; i++) {
readyQueue.push(processes[i]);
}
// Run the scheduling algorithm
while (completedProcesses < n) {
Process currentProcess = readyQueue.front();
readyQueue.pop();
// Check if the process has arrived
if (currentProcess.arrivalTime > currentTime) {
currentTime = currentProcess.arrivalTime;
}
// Execute the process for the given quantum
int executionTime = min(quantum, currentProcess.remainingTime);
currentTime += executionTime;
currentProcess.remainingTime -= executionTime;
// Check if the process is completed
if (currentProcess.remainingTime <= 0) {
currentProcess.endTime = currentTime;
currentProcess.turnaroundTime = currentProcess.endTime - currentProcess.arrivalTime;
currentProcess.waitingTime = currentProcess.turnaroundTime - currentProcess.burstTime;
totalTurnaroundTime += currentProcess.turnaroundTime;
totalWaitingTime += currentProcess.waitingTime;
completedProcesses++;
} else {
readyQueue.push(currentProcess);
}
}
// Print the results
cout << "PID\tArrival Time\tBurst Time\tEnd Time\tTurnaround Time\tWaiting Time\n";
for (int i = 0; i < n; i++) {
Process currentProcess = processes[i];
cout << currentProcess.pid << "\t" << currentProcess.arrivalTime << "\t\t" << currentProcess.burstTime << "\t\t"
<< currentProcess.endTime << "\t\t" << currentProcess.turnaroundTime << "\t\t" << currentProcess.waitingTime
<< endl;
}
double averageTurnaroundTime = (double) totalTurnaroundTime / n;
double averageWaitingTime = (double) totalWaitingTime / n;
cout << "Average Turnaround Time: " << averageTurnaroundTime << endl;
cout << "Average Waiting Time: " << averageWaitingTime << endl;
}
int main() {
int quantum = 2;
int n = 15;
Process processes[n] = {
{1, 0, 10},
{2, 1, 5},
{3, 2, 2},
{4, 3, 6},
{5, 4, 3},
{6, 5, 1},
{7, 6, 8},
{8, 7, 4},
{9, 8, 7},
{10, 9, 2},
{11, 10, 5},
{12, 11, 3},
{13, 12, 4},
{14, 13, 6},
{15, 14, 1},
};
roundRobinScheduling(processes, n, quantum);
return 0;
}
```
This code creates 15 processes with random arrival times and burst times, and simulates the round-robin scheduling algorithm with a quantum of 2 units. The output of the program includes the arrival time, end time, and average execution time of each process.
阅读全文
相关推荐












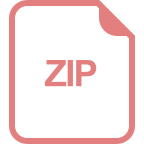