Write the code in C++.1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to the terminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time.
时间: 2023-12-06 20:44:12 浏览: 142
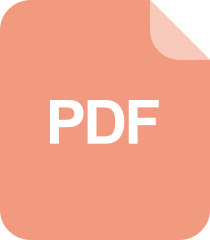
algorithms in C++

Here's the code in C++ for simulating the round-robin algorithm for process scheduling:
```
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
struct Process {
int pid; // process id
int arrival_time; // arrival time of process
int burst_time; // burst time of process
int remaining_time; // remaining time of the process
int end_time; // end time of the process
};
void roundRobin(vector<Process>& processes, int quantum) {
int n = processes.size();
queue<int> q; // queue to store the process indexes
int t = 0; // current time
int total_burst_time = 0; // total burst time of all processes
int completed_processes = 0; // number of completed processes
int waiting_time = 0; // total waiting time of all processes
// initialize remaining time of all processes as their burst time
for (int i = 0; i < n; i++) {
processes[i].remaining_time = processes[i].burst_time;
total_burst_time += processes[i].burst_time;
}
// run the scheduler until all processes are completed
while (completed_processes < n) {
// add processes to the queue that have arrived by the current time
for (int i = 0; i < n; i++) {
if (processes[i].arrival_time <= t && processes[i].remaining_time > 0) {
q.push(i);
}
}
// if no processes are in the queue, increment time
if (q.empty()) {
t++;
continue;
}
// get the next process from the queue
int curr = q.front();
q.pop();
// execute the process for the quantum time or until completion
int execution_time = min(processes[curr].remaining_time, quantum);
processes[curr].remaining_time -= execution_time;
t += execution_time;
// add the process back to the queue if it still has remaining time
if (processes[curr].remaining_time > 0) {
q.push(curr);
} else {
// process has completed, update completion time and waiting time
completed_processes++;
processes[curr].end_time = t;
waiting_time += (t - processes[curr].arrival_time - processes[curr].burst_time);
}
}
// calculate average execution time and waiting time
float avg_execution_time = (float) total_burst_time / n;
float avg_waiting_time = (float) waiting_time / n;
// output scheduling situation to terminal
cout << "PID\tArrival Time\tBurst Time\tEnd Time" << endl;
for (int i = 0; i < n; i++) {
cout << processes[i].pid << "\t" << processes[i].arrival_time << "\t\t" << processes[i].burst_time << "\t\t";
if (processes[i].end_time == 0) {
cout << "N/A" << endl;
} else {
cout << processes[i].end_time << endl;
}
}
cout << "Average Execution Time: " << avg_execution_time << endl;
cout << "Average Waiting Time: " << avg_waiting_time << endl;
}
int main() {
vector<Process> processes = {{1, 0, 5}, {2, 1, 7}, {3, 2, 3}, {4, 3, 4}, {5, 4, 2}, {6, 5, 6}, {7, 6, 8}, {8, 7, 1}, {9, 8, 5}, {10, 9, 3}, {11, 10, 6}, {12, 11, 2}, {13, 12, 4}, {14, 13, 5}, {15, 14, 2}};
int quantum = 2;
roundRobin(processes, quantum);
return 0;
}
```
This code simulates the round-robin algorithm for process scheduling for a set of 15 processes with different arrival times and burst times. The output includes the arrival time, burst time, and end time of each process, as well as the average execution time and average waiting time of all processes. You can adjust the values of the `processes` and `quantum` variables to test the algorithm with different sets of processes and quantum values.
阅读全文
相关推荐
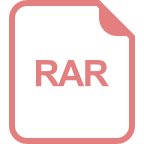
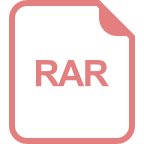








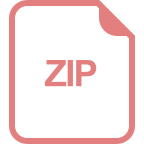
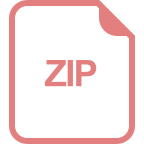
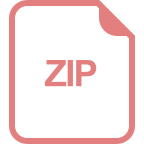
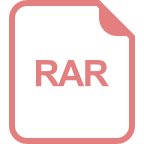
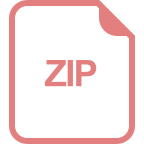