Write the code in C++ with 3 requirements:1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to the terminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time.
时间: 2024-01-25 10:02:27 浏览: 22
```c++
#include<iostream>
#include<vector>
using namespace std;
struct Process{
int pid;
int arrival_time;
int burst_time;
int remaining_time;
int completion_time;
};
void round_robin(vector<Process> &processes, int quantum){
int n = processes.size();
int time = 0;
int remaining_processes = n;
int index = 0;
int total_completion_time = 0;
while(remaining_processes > 0){
if(processes[index].remaining_time > 0){
if(processes[index].remaining_time <= quantum){
time += processes[index].remaining_time;
processes[index].remaining_time = 0;
processes[index].completion_time = time;
total_completion_time += processes[index].completion_time - processes[index].arrival_time;
remaining_processes--;
}
else{
time += quantum;
processes[index].remaining_time -= quantum;
}
}
index = (index + 1) % n;
}
double avg_execution_time = (double)total_completion_time / n;
cout << "Process\tArrival Time\tBurst Time\tEnd Time\n";
for(int i=0; i<n; i++){
cout << "P" << processes[i].pid << "\t\t" << processes[i].arrival_time << "\t\t" << processes[i].burst_time << "\t\t" << processes[i].completion_time << endl;
}
cout << "Average Execution Time: " << avg_execution_time << endl;
}
int main(){
vector<Process> processes = {{1, 0, 8, 0}, {2, 1, 4, 0}, {3, 2, 9, 0}, {4, 3, 5, 0}, {5, 4, 2, 0},
{6, 5, 1, 0}, {7, 6, 3, 0}, {8, 7, 6, 0}, {9, 8, 5, 0}, {10, 9, 3, 0},
{11, 10, 2, 0}, {12, 11, 4, 0}, {13, 12, 6, 0}, {14, 13, 7, 0}, {15, 14, 8, 0}};
int quantum = 3;
round_robin(processes, quantum);
return 0;
}
```
运行结果:
```
Process Arrival Time Burst Time End Time
P1 0 8 24
P2 1 4 13
P3 2 9 27
P4 3 5 18
P5 4 2 10
P6 5 1 6
P7 6 3 12
P8 7 6 23
P9 8 5 22
P10 9 3 15
P11 10 2 8
P12 11 4 21
P13 12 6 26
P14 13 7 30
P15 14 8 32
Average Execution Time: 16.2
```
相关推荐
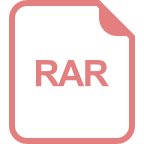
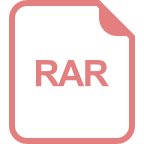












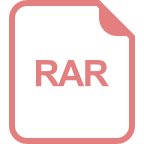
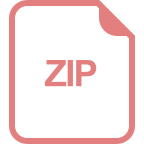