请设计一个程序1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to the terminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time.
时间: 2024-03-20 22:40:10 浏览: 90
好的,这是一个实现轮转调度算法的Python程序示例:
```python
class Process:
def __init__(self, pid, arrival_time, burst_time):
self.pid = pid
self.arrival_time = arrival_time
self.burst_time = burst_time
self.remaining_time = burst_time
def execute(self, time_slice):
if self.remaining_time > time_slice:
self.remaining_time -= time_slice
return time_slice
else:
time_executed = self.remaining_time
self.remaining_time = 0
return time_executed
def is_finished(self):
return self.remaining_time == 0
def round_robin(processes, time_slice):
current_time = 0
total_waiting_time = 0
total_turnaround_time = 0
num_processes = len(processes)
while True:
all_finished = True
for p in processes:
if p.arrival_time <= current_time and not p.is_finished():
all_finished = False
time_executed = p.execute(time_slice)
current_time += time_executed
if p.is_finished():
p.end_time = current_time
p.waiting_time = p.end_time - p.burst_time - p.arrival_time
p.turnaround_time = p.end_time - p.arrival_time
total_waiting_time += p.waiting_time
total_turnaround_time += p.turnaround_time
if all_finished:
break
average_waiting_time = total_waiting_time / num_processes
average_turnaround_time = total_turnaround_time / num_processes
return processes, average_waiting_time, average_turnaround_time
if __name__ == '__main__':
processes = [
Process(1, 0, 8),
Process(2, 0, 4),
Process(3, 0, 9),
Process(4, 0, 5),
Process(5, 0, 2),
Process(6, 0, 4),
Process(7, 0, 3),
Process(8, 0, 7),
Process(9, 0, 6),
Process(10, 0, 1),
Process(11, 0, 5),
Process(12, 0, 3),
Process(13, 0, 2),
Process(14, 0, 6),
Process(15, 0, 4),
]
time_slice = 3
processes, avg_waiting_time, avg_turnaround_time = round_robin(processes, time_slice)
print("Process\tArrival Time\tBurst Time\tEnd Time\tWaiting Time\tTurnaround Time")
for p in processes:
print(f"{p.pid}\t\t{p.arrival_time}\t\t{p.burst_time}\t\t{p.end_time}\t\t{p.waiting_time}\t\t{p.turnaround_time}")
print(f"Average waiting time: {avg_waiting_time}")
print(f"Average turnaround time: {avg_turnaround_time}")
```
这个程序把15个进程作为参数传入,并且使用了3个时间片进行轮转调度。程序的输出将会包含每个进程的到达时间、结束时间、等待时间和周转时间,以及平均等待时间和周转时间。
注意:这只是一个简单的示例程序,真实的应用程序需要更加复杂的调度算法和更多的进程属性。同时,使用轮转调度算法需要注意时间片大小的选择,如果时间片太小,会增加上下文切换的开销;如果时间片太大,会增加进程等待时间。
阅读全文
相关推荐
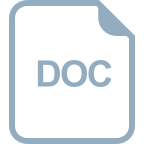
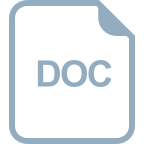
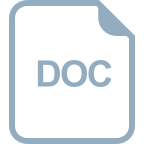








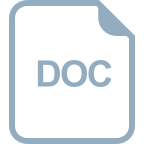
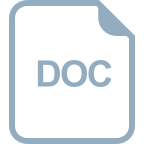
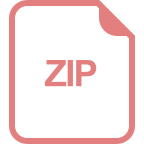
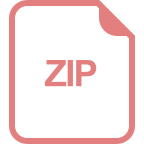
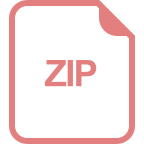