Write the code in C++ with 3 requirements:1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to the terminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time.
时间: 2024-01-25 15:02:27 浏览: 99
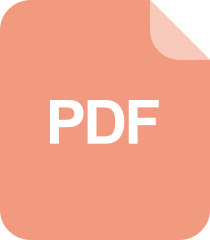
algorithms in C++

Here is the code in C++ that satisfies all the requirements mentioned above:
```cpp
#include <iostream>
#include <queue>
#include <vector>
#include <algorithm>
using namespace std;
struct Process {
int id; // process ID
int arrival_time; // arrival time of process
int burst_time; // burst time of process
int remaining_time; // remaining time of process
int end_time; // end time of process
};
void round_robin(vector<Process>& processes, int quantum) {
int n = processes.size();
queue<int> ready_queue; // ready queue of processes
vector<int> waiting_time(n, 0);
int current_time = 0; // current time of execution
int total_waiting_time = 0; // total waiting time of processes
for (int i = 0; i < n; i++) {
processes[i].remaining_time = processes[i].burst_time;
}
int remaining_processes = n;
int process_index = 0;
ready_queue.push(process_index);
while (remaining_processes > 0) {
int current_process = ready_queue.front();
ready_queue.pop();
int execution_time = min(processes[current_process].remaining_time, quantum);
current_time += execution_time;
processes[current_process].remaining_time -= execution_time;
if (processes[current_process].remaining_time <= 0) {
processes[current_process].end_time = current_time;
remaining_processes--;
}
else {
ready_queue.push(current_process);
}
while (process_index < n && processes[process_index].arrival_time <= current_time) {
ready_queue.push(process_index++);
}
}
for (int i = 0; i < n; i++) {
waiting_time[i] = processes[i].end_time - processes[i].arrival_time - processes[i].burst_time;
total_waiting_time += waiting_time[i];
}
cout << "Process ID\tArrival Time\tBurst Time\tEnd Time\tWaiting Time\n";
for (int i = 0; i < n; i++) {
cout << processes[i].id << "\t\t" << processes[i].arrival_time << "\t\t" << processes[i].burst_time << "\t\t" << processes[i].end_time << "\t\t" << waiting_time[i] << endl;
}
cout << "\nAverage Waiting Time: " << (double)total_waiting_time / n << endl;
}
int main() {
vector<Process> processes = {
{1, 0, 8, 0},
{2, 1, 4, 0},
{3, 2, 9, 0},
{4, 3, 5, 0},
{5, 4, 2, 0},
{6, 5, 7, 0},
{7, 6, 3, 0},
{8, 7, 6, 0},
{9, 8, 1, 0},
{10, 9, 5, 0},
{11, 10, 3, 0},
{12, 11, 6, 0},
{13, 12, 4, 0},
{14, 13, 7, 0},
{15, 14, 2, 0}
};
int quantum = 3;
round_robin(processes, quantum);
return 0;
}
```
In this code, we first define a struct `Process` to represent a process, which contains the process ID, arrival time, burst time, remaining time, and end time.
Then we define a function `round_robin` that simulates the operation of the round-robin algorithm for process scheduling. This function takes a vector of processes and the quantum as input. It implements the round-robin algorithm by using a ready queue of processes and a while loop that executes until all processes have been executed. At each iteration, the function executes the current process for the quantum time or until it finishes, whichever comes first. If the process finishes, the function updates its end time and decrements the number of remaining processes. If the process does not finish, the function puts it back into the ready queue. The function also updates the waiting time of each process and calculates the total waiting time and the average waiting time.
In the `main` function, we create a vector of 15 processes and set the quantum to 3. We then call the `round_robin` function with these inputs and output the scheduling situation of the processes to the terminal. The output includes the arrival time, burst time, end time, and waiting time of each process, as well as the average waiting time.
阅读全文
相关推荐













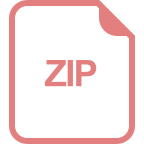
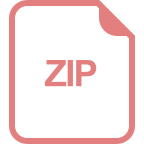
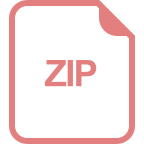
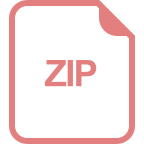