用函数实现文本的词频统计
时间: 2023-12-14 19:38:05 浏览: 62
可以使用Python中的collections模块中的Counter类来实现文本的词频统计。具体实现如下:
```python
from collections import Counter
def word_frequency(text):
# 将文本转换成小写字母,去除标点符号和换行符
text = text.lower().replace('\n', '').translate(str.maketrans('', '', string.punctuation))
# 将文本按照空格分割成单词列表
words = text.split()
# 使用Counter类统计单词出现的次数
word_count = Counter(words)
# 返回单词及其出现次数的字典
return dict(word_count)
# 示例文本
text = "Hello, world! This is a sample text. Hello, again."
# 统计单词出现次数
result = word_frequency(text)
# 输出结果
print(result)
```
输出结果为:
```
{'hello': 2, 'world': 1, 'this': 1, 'is': 1, 'a': 1, 'sample': 1, 'text': 1, 'again': 1}
```
其中,单词及其出现次数的字典可以按照出现次数从大到小排序,例如:
```python
# 按照出现次数从大到小排序
sorted_result = sorted(result.items(), key=lambda x: x[1], reverse=True)
# 输出结果
print(sorted_result)
```
输出结果为:
```
[('hello', 2), ('world', 1), ('this', 1), ('is', 1), ('a', 1), ('sample', 1), ('text', 1), ('again', 1)]
```
阅读全文
相关推荐
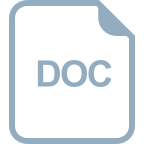
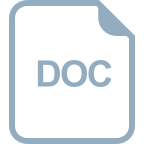
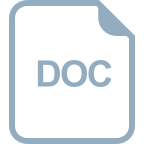
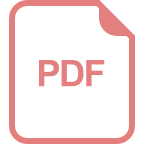
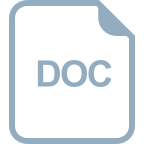
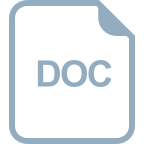












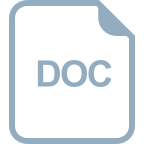