文本词频统计,Hamlet英文词频统计以及《三国演义》人物出场统计
时间: 2024-03-14 10:47:31 浏览: 74
1. 文本词频统计
以下是一个简单的 Python 代码示例,用于统计给定文本文件中每个单词出现的次数:
```python
import re
def word_count(file_path):
with open(file_path, 'r') as f:
text = f.read()
words = re.findall(r'\b\w+\b', text.lower())
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
# 测试代码
file_path = 'test.txt'
print(word_count(file_path))
```
需要注意的是,此代码示例使用正则表达式来匹配单词,因此可以处理包括带有连字符的单词在内的多种文本格式。
2. Hamlet英文词频统计
以下是一个使用Python代码的Hamlet英文词频统计示例:
```python
import re
def word_count(file_path):
with open(file_path, 'r') as f:
text = f.read()
words = re.findall(r'\b\w+\b', text.lower())
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
# 测试代码
file_path = 'hamlet.txt'
word_count = word_count(file_path)
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
for word, count in sorted_word_count[:10]:
print(word, count)
```
此代码示例首先使用与之前的示例相同的方法来统计Hamlet文本文件中每个单词的出现次数。然后,它使用Python的`sorted()`函数按出现次数对单词进行排序,并输出前10个最常出现的单词及其出现次数。
3.《三国演义》人物出场统计
以下是一个简单的 Python 代码示例,用于统计《三国演义》中每个人物出现的次数:
```python
import re
def character_count(file_path):
with open(file_path, 'r') as f:
text = f.read()
characters = re.findall(r'[^\u4e00-\u9fff\s]+', text)
character_count = {}
for character in characters:
if character in character_count:
character_count[character] += 1
else:
character_count[character] = 1
return character_count
# 测试代码
file_path = 'sanguoyanyi.txt'
character_count = character_count(file_path)
sorted_character_count = sorted(character_count.items(), key=lambda x: x[1], reverse=True)
for character, count in sorted_character_count[:10]:
print(character, count)
```
此代码示例使用正则表达式来匹配所有非中文字符,从而提取出《三国演义》中的所有人物。然后,它使用Python的`sorted()`函数按出现次数对人物进行排序,并输出前10个出现次数最多的人物及其出现次数。需要注意的是,此代码示例仅适用于文本文件中人物名称用非中文字符分隔的情况。如果人物名称以中文字符出现,则需要使用其他方法进行处理。
阅读全文
相关推荐
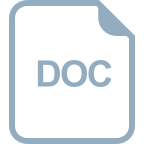
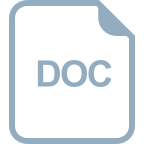
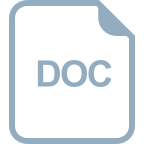
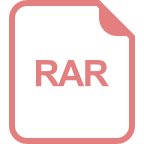






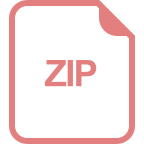
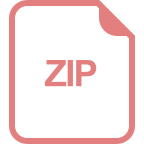
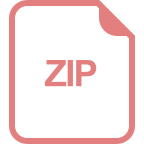
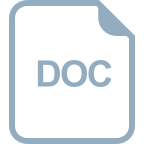
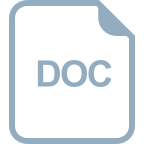
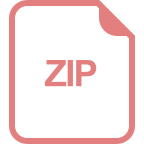
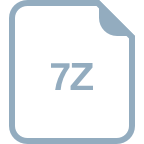
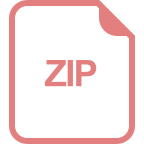
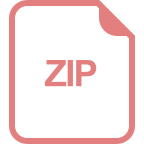
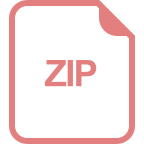