如何用c++获取temp文件夹
时间: 2024-05-09 19:21:22 浏览: 58
在C语言中,可以使用以下代码获取系统的临时文件夹路径:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char* temp_dir = getenv("TMPDIR");
if (temp_dir == NULL) {
temp_dir = getenv("TMP");
if (temp_dir == NULL) {
temp_dir = getenv("TEMP");
if (temp_dir == NULL) {
temp_dir = "/tmp"; // 如果上述环境变量都不存在,则默认为 /tmp
}
}
}
printf("Temporary directory: %s\n", temp_dir);
return 0;
}
```
该代码会依次检查环境变量 `TMPDIR`、`TMP` 和 `TEMP` 是否存在,如果存在则返回变量值,否则返回 `/tmp`。注意,在不同的操作系统中,临时文件夹的路径可能不同,因此建议使用上述方式获取临时文件夹路径。
相关问题
C++实现将文件剪切到temp文件夹里
在C++中,你可以使用标准库中的`fstream`和`filesystem`模块来操作文件,包括剪切文件到另一个位置。这里是一个基本的例子,展示如何将文件剪切(实际上是移动)到指定的临时目录(如`temp`文件夹):
```cpp
#include <iostream>
#include <fstream>
#include <filesystem>
namespace fs = std::filesystem;
void copy_file_to_temp(const std::string& source_path, const std::string& target_dir) {
// 检查目标目录是否存在,不存在则创建
if (!fs::exists(target_dir)) {
fs::create_directory(target_dir);
}
// 获取源文件名,以便构建新路径
std::string filename = fs::path(source_path).filename().string();
std::string target_file = fs::path(target_dir) / filename; // 构建新的完整目标路径
// 使用fstream进行移动操作
std::ifstream in(source_path, std::ios::binary);
std::ofstream out(target_file, std::ios::binary);
// 如果所有操作都成功,则复制内容并关闭文件流
if (in.is_open() && out.is_open()) {
out << in.rdbuf();
in.close();
out.close();
std::cout << "File moved successfully from " << source_path << " to " << target_file << "\n";
} else {
std::cerr << "Error: Unable to move file!" << std::endl;
}
}
int main() {
try {
// 替换为你想要剪切的实际文件路径
copy_file_to_temp("source.txt", "temp");
} catch (const std::exception& e) {
std::cerr << "An error occurred: " << e.what() << std::endl;
}
return 0;
}
```
C++实现将文件移动到temp文件夹里
在C++中,你可以使用C++标准库`<filesystem>`来实现文件的移动功能。下面是一个简单的示例,演示如何将文件从给定源路径移动到系统的临时文件夹(通常位于`"/tmp"`目录下):
```cpp
#include <iostream>
#include <filesystem>
void move_file_to_temp(const std::string& src_path, const std::string& temp_dir_path) {
// 检查临时目录是否存在,不存在则创建
if (!std::filesystem::exists(temp_dir_path)) {
try {
std::filesystem::create_directory(temp_dir_path);
std::cout << "临时目录创建成功:" << temp_dir_path << '\n';
} catch (const std::filesystem::filesystem_error& e) {
std::cerr << "创建临时目录失败: " << e.what() << '\n';
return;
}
}
// 获取文件名以便于新路径构建
auto file_name = std::filesystem::path(src_path).filename();
// 完整的目标路径
std::string target_path = temp_dir_path + "/" + file_name;
// 检查源文件是否存在并移动
if (std::filesystem::exists(src_path)) {
try {
std::filesystem::rename(src_path, target_path);
std::cout << "文件" << src_path << "已移动至:" << target_path << '\n';
} catch (const std::filesystem::filesystem_error& e) {
std::cerr << "移动文件失败: " << e.what() << '\n';
}
} else {
std::cerr << "源文件" << src_path << "不存在,无法移动.\n";
}
}
int main() {
// 调用函数传入具体的源文件路径和临时文件夹路径
move_file_to_temp("path_to_your_file.txt", "/tmp");
return 0;
}
```
这个例子假设`move_file_to_temp`函数接受两个字符串参数,分别代表源文件路径和临时目录路径。如果一切顺利,文件会被移动;如果遇到任何错误,会捕获异常并打印错误信息。
阅读全文
相关推荐
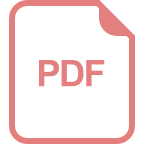
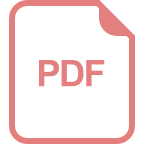
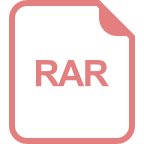


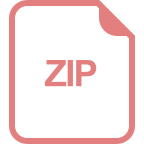
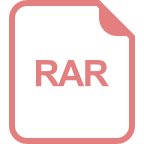
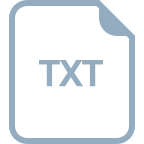
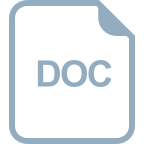
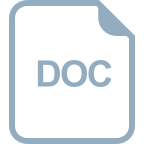
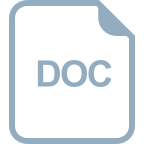
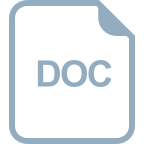


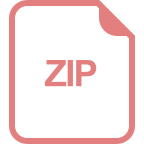