将上面程序合并为一个程序文件
时间: 2024-06-09 11:08:03 浏览: 16
以下是将两个程序合并为一个程序文件的代码:
```python
import openai
import requests
import json
# OpenAI API credentials
openai.api_key = "YOUR_API_KEY"
# CSDN API credentials
csdn_app_key = "YOUR_APP_KEY"
csdn_app_secret = "YOUR_APP_SECRET"
csdn_username = "YOUR_USERNAME"
csdn_password = "YOUR_PASSWORD"
# Authenticate CSDN API
response = requests.post(
url="https://passport.csdn.net/v1/api/authorize",
params={
"client_id": csdn_app_key,
"response_type": "code",
"state": "random_string",
"redirect_uri": "http://localhost",
"display": "default"
},
headers={
"Content-Type": "application/json",
"User-Agent": "Mozilla/5.0"
},
auth=(csdn_username, csdn_password)
)
auth_code = json.loads(response.text)['data']['auth_code']
# Use auth code to get access token
response = requests.post(
url="https://gitee.com/oauth/token",
params={
"grant_type": "authorization_code",
"code": auth_code,
"client_id": csdn_app_key,
"redirect_uri": "http://localhost",
"client_secret": csdn_app_secret
},
headers={
"Content-Type": "application/json",
"User-Agent": "Mozilla/5.0"
}
)
access_token = json.loads(response.text)['access_token']
# Chat with OpenAI GPT-3
def chat_with_openai(prompt):
response = openai.Completion.create(
engine="davinci",
prompt=prompt,
max_tokens=150,
n=1,
stop=None,
temperature=0.5
)
message = response.choices[0].text
return message.strip()
# Chat with CSDN ChitGPT
def chat_with_csdn(prompt):
response = requests.post(
url="https://aiapi.csdn.net/sdk/chit_chat",
headers={
"Content-Type": "application/json",
"User-Agent": "Mozilla/5.0",
"Authorization": f"Bearer {access_token}"
},
json={
"msg": prompt
}
)
message = json.loads(response.text)['data']['reply']
return message.strip()
# Main loop
while True:
user_input = input("You: ")
if user_input.lower() == "bye":
print("Bot: Bye!")
break
openai_response = chat_with_openai(user_input)
print("OpenAI: " + openai_response)
csdn_response = chat_with_csdn(user_input)
print("CSDN: " + csdn_response)
```
请注意,您需要将 `YOUR_API_KEY`,`YOUR_APP_KEY`,`YOUR_APP_SECRET`,`YOUR_USERNAME` 和 `YOUR_PASSWORD` 替换为您自己的 API 密钥和凭据。
相关推荐
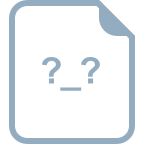














