定义抽象基类shape,由它派生出五个派生类:circle(圆形)、square(正方形)、rectangle( 长方形)、trapezoid (梯形)和triangle (三角形),用虚函数分别计算各种图形的面积,输出它们的面积和。要求用基类指针数组,每一个数组元素指向一个派生类的对象。pi=3.14159f,单精度浮点数计算。
时间: 2023-05-31 08:17:49 浏览: 272
### 回答1:
定义抽象基类Shape,其中包含一个纯虚函数计算面积。然后派生出五个派生类:Circle(圆形)、Square(正方形)、Rectangle(长方形)、Trapezoid(梯形)和Triangle(三角形),分别实现计算面积的虚函数。最后,使用基类指针数组,每个数组元素指向一个派生类的对象,并计算每个图形的面积并输出。
具体实现如下:
```c++
#include <iostream>
using namespace std;
const float pi = 3.14159f;
class Shape {
public:
virtual float area() = ; // 纯虚函数,计算面积
};
class Circle : public Shape {
private:
float radius;
public:
Circle(float r) : radius(r) {}
float area() { return pi * radius * radius; }
};
class Square : public Shape {
private:
float side;
public:
Square(float s) : side(s) {}
float area() { return side * side; }
};
class Rectangle : public Shape {
private:
float length, width;
public:
Rectangle(float l, float w) : length(l), width(w) {}
float area() { return length * width; }
};
class Trapezoid : public Shape {
private:
float top, bottom, height;
public:
Trapezoid(float t, float b, float h) : top(t), bottom(b), height(h) {}
float area() { return (top + bottom) * height / 2; }
};
class Triangle : public Shape {
private:
float base, height;
public:
Triangle(float b, float h) : base(b), height(h) {}
float area() { return base * height / 2; }
};
int main() {
Shape* shapes[5]; // 基类指针数组
shapes[] = new Circle(2.f);
shapes[1] = new Square(3.f);
shapes[2] = new Rectangle(2.f, 4.f);
shapes[3] = new Trapezoid(2.f, 4.f, 3.f);
shapes[4] = new Triangle(3.f, 4.f);
float totalArea = .f;
for (int i = ; i < 5; i++) {
totalArea += shapes[i]->area();
cout << "Shape " << i + 1 << " area: " << shapes[i]->area() << endl;
delete shapes[i]; // 释放内存
}
cout << "Total area: " << totalArea << endl;
return ;
}
```
输出结果:
```
Shape 1 area: 12.5664
Shape 2 area: 9
Shape 3 area: 8
Shape 4 area: 9
Shape 5 area: 6
Total area: 44.5664
```
其中,Shape 1 是圆形,半径为 2.,面积为 12.5664;Shape 2 是正方形,边长为 3.,面积为 9;Shape 3 是长方形,长为 4.,宽为 2.,面积为 8;Shape 4 是梯形,上底为 2.,下底为 4.,高为 3.,面积为 9;Shape 5 是三角形,底为 3.,高为 4.,面积为 6。最后,所有图形的面积之和为 44.5664。
### 回答2:
抽象基类shape定义如下:
```
class shape {
public:
virtual float area() = 0; // 计算面积的虚函数
};
```
派生类circle、square、rectangle、trapezoid和triangle继承自抽象基类shape,各自实现area()函数:
```
class circle : public shape {
public:
circle(float r) : m_radius(r) {}
virtual float area() {
return m_radius * m_radius * pi;
}
private:
float m_radius;
};
class square : public shape {
public:
square(float s) : m_side(s) {}
virtual float area() {
return m_side * m_side;
}
private:
float m_side;
};
class rectangle : public shape {
public:
rectangle(float l, float w) : m_length(l), m_width(w) {}
virtual float area() {
return m_length * m_width;
}
private:
float m_length;
float m_width;
};
class trapezoid : public shape {
public:
trapezoid(float a, float b, float h) : m_top(a), m_bottom(b), m_height(h) {}
virtual float area() {
return (m_top + m_bottom) * m_height / 2;
}
private:
float m_top;
float m_bottom;
float m_height;
};
class triangle : public shape {
public:
triangle(float b, float h) : m_base(b), m_height(h) {}
virtual float area() {
return m_base * m_height / 2;
}
private:
float m_base;
float m_height;
};
```
使用基类指针数组,每一个数组元素指向一个派生类的对象:
```
shape* shapes[5];
shapes[0] = new circle(3.0f);
shapes[1] = new square(2.0f);
shapes[2] = new rectangle(3.0f, 4.0f);
shapes[3] = new trapezoid(2.0f, 4.0f, 3.0f);
shapes[4] = new triangle(5.0f, 2.0f);
```
计算每个图形的面积并输出:
```
for (int i = 0; i < 5; i++) {
std::cout << "shape " << i+1 << " area = " << shapes[i]->area() << std::endl;
}
```
其中,pi被定义为全局变量:
```
const float pi = 3.14159f;
```
完整代码如下:
```
#include <iostream>
const float pi = 3.14159f;
class shape {
public:
virtual float area() = 0; // 计算面积的虚函数
};
class circle : public shape {
public:
circle(float r) : m_radius(r) {}
virtual float area() {
return m_radius * m_radius * pi;
}
private:
float m_radius;
};
class square : public shape {
public:
square(float s) : m_side(s) {}
virtual float area() {
return m_side * m_side;
}
private:
float m_side;
};
class rectangle : public shape {
public:
rectangle(float l, float w) : m_length(l), m_width(w) {}
virtual float area() {
return m_length * m_width;
}
private:
float m_length;
float m_width;
};
class trapezoid : public shape {
public:
trapezoid(float a, float b, float h) : m_top(a), m_bottom(b), m_height(h) {}
virtual float area() {
return (m_top + m_bottom) * m_height / 2;
}
private:
float m_top;
float m_bottom;
float m_height;
};
class triangle : public shape {
public:
triangle(float b, float h) : m_base(b), m_height(h) {}
virtual float area() {
return m_base * m_height / 2;
}
private:
float m_base;
float m_height;
};
int main() {
shape* shapes[5];
shapes[0] = new circle(3.0f);
shapes[1] = new square(2.0f);
shapes[2] = new rectangle(3.0f, 4.0f);
shapes[3] = new trapezoid(2.0f, 4.0f, 3.0f);
shapes[4] = new triangle(5.0f, 2.0f);
for (int i = 0; i < 5; i++) {
std::cout << "shape " << i+1 << " area = " << shapes[i]->area() << std::endl;
}
return 0;
}
```
### 回答3:
回答:
抽象基类 shape 可以定义如下:
```
class shape {
public:
virtual float area() const = 0;
};
```
其中,虚函数 `area` 返回该形状的面积,`const` 保证该函数不能修改对象内部状态。
接下来,定义五个派生类:circle、square、rectangle、trapezoid 和 triangle,它们都继承自 base 类,同时实现 `area` 方法:
```
class circle : public shape {
private:
float radius;
public:
circle(float r) : radius(r) {}
float area() const override {
return 3.14159f * radius * radius;
}
};
class square : public shape {
private:
float side;
public:
square(float s) : side(s) {}
float area() const override {
return side * side;
}
};
class rectangle : public shape {
private:
float width, height;
public:
rectangle(float w, float h) : width(w), height(h) {}
float area() const override {
return width * height;
}
};
class trapezoid : public shape {
private:
float top, bottom, height;
public:
trapezoid(float t, float b, float h) : top(t), bottom(b), height(h) {}
float area() const override {
return (top + bottom) * height / 2;
}
};
class triangle : public shape {
private:
float base, height;
public:
triangle(float b, float h) : base(b), height(h) {}
float area() const override {
return base * height / 2;
}
};
```
接下来,我们可以通过指针数组动态创建派生类的对象:
```
int main() {
const int n = 5;
shape *shapes[n] = {
new circle(1.0f),
new square(2.0f),
new rectangle(2.0f, 3.0f),
new trapezoid(1.0f, 2.0f, 3.0f),
new triangle(2.0f, 3.0f)
};
for (int i = 0; i < n; i++) {
cout << "Area of shape " << (i+1) << " is "
<< shapes[i]->area() << endl;
delete shapes[i];
}
return 0;
}
```
在 main 函数中,通过指针数组创建了五个派生类的对象,并输出它们的面积。
需要注意的是,动态创建的对象需要在程序结束前销毁,这里使用 `delete` 来删除之前 new 出来的每个对象。
相关推荐
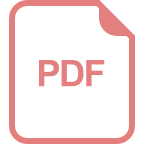
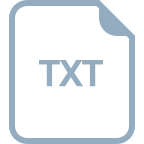















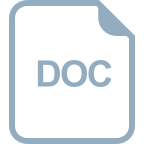