ip5306 iic 代码
时间: 2025-01-03 19:25:30 浏览: 5
### IP5306 IIC Communication Code Example
For the IP5306 chip, which is commonly used in power management systems for devices like smartphones and tablets, implementing an I2C (Inter-Integrated Circuit) communication protocol allows interaction with this device from a microcontroller or processor. Below demonstrates how to write C code that interfaces with the IP5306 using Linux's I2C framework.
#### Initialization of I2C Adapter
The initialization process involves setting up the adapter responsible for managing transactions over the bus.
```c
#include <linux/i2c.h>
#include <linux/module.h>
static int ip5306_i2c_probe(struct i2c_client *client, const struct i2c_device_id *id)
{
// Check if the connected client matches expected properties
if (!i2c_check_functionality(client->adapter, I2C_FUNC_I2C)) {
dev_err(&client->dev, "I2C functionality not supported\n");
return -ENODEV;
}
// Initialize hardware-specific settings here...
dev_info(&client->dev, "IP5306 Device Detected at address %d!\n", client->addr);
return 0; // Success
}
```
This function checks whether the required functionalities are available on the given `adapter` before proceeding further[^4].
#### Register Read Function
To read data from registers within the IP5306, one can implement a helper function as follows:
```c
int ip5306_read_reg(struct i2c_client *client, u8 reg, u8 *val)
{
union i2c_smbus_data data;
if (i2c_smbus_xfer(client->adapter, client->addr,
client->flags & I2C_M_TEN,
I2C_SMBUS_READ, reg,
I2C_SMBUS_BYTE_DATA, &data))
return -EFAULT;
*val = data.byte;
return 0;
}
```
Here, `i2c_smbus_xfer()` performs the actual transaction by sending out commands through the specified `adapter`, targeting the specific register (`reg`) inside the IP5306 chip.
#### Register Write Function
Similarly, writing values into these registers requires another utility method:
```c
int ip5306_write_reg(struct i2c_client *client, u8 reg, u8 val)
{
union i2c_smbus_data data;
data.byte = val;
if (i2c_smbus_xfer(client->adapter, client->addr,
client->flags & I2C_M_TEN,
I2C_SMBUS_WRITE, reg,
I2C_SMBUS_BYTE_DATA, &data))
return -EFAULT;
return 0;
}
```
These functions facilitate direct manipulation of internal states via software control while ensuring proper synchronization between master-slave communications.
--related questions--
1. How does the IP5306 handle different types of interrupts during operation?
2. What measures should be taken when debugging issues related to I2C communication failures?
3. Can you explain more about configuring GPIO pins associated with the IP5306 module?
4. In what scenarios would someone prefer SPI over I2C for interfacing with similar ICs?
阅读全文
相关推荐






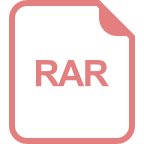
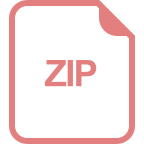

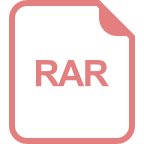









