func GetReportList(db *sql.DB, data *model.ReportReq) (int, string, string, string, string, string, string, string, string, string, string, string, string) { var Report_Num, Report_Name, Hospital_Id, Hospital_Name, User_Id, Name, Gender, Age, Phone, Createtime_String, Report_Image string sqlStr := "select report_num,report_name,hospital_id,hospital_name,user_id,name,gender,age,phone,createtime_string,report_image from report where name = ?" err := db.QueryRow(sqlStr, data.Name).Scan( &Report_Num, &Report_Name, &Hospital_Id, &Hospital_Name, &User_Id, &Name, &Gender, &Age, &Phone, &Createtime_String, &Report_Image) if err != nil { logger.Sugar.Info(err) return 0, "", "", "", "", "", "", "", "", "", "", "", "" } return 200, "登陆成功", Report_Num, Report_Name, Hospital_Id, Hospital_Name, User_Id, Name, Gender, Age, Phone, Createtime_String, Report_Image } 帮我看看哪里错了
时间: 2023-07-31 17:06:15 浏览: 60
这段代码的问题应该出在SQL查询语句上。你的SQL语句中只查询了 `name` 这一列,而在 `Scan` 方法中又按顺序扫描了所有列的值。这会导致在扫描到第一个不存在的列时出现错误,进而导致整个查询失败。你应该在查询语句中查询所有需要的列,例如:
```
sqlStr := "select report_num,report_name,hospital_id,hospital_name,user_id,name,gender,age,phone,createtime_string,report_image from report where name = ?"
```
同时,你也应该确保查询到的行数只有一行,否则会出现无法处理的情况。你可以通过给查询语句添加限制条件来保证只查询一行,例如:
```
sqlStr := "select report_num,report_name,hospital_id,hospital_name,user_id,name,gender,age,phone,createtime_string,report_image from report where name = ? limit 1"
```
最后,你的返回值中,第一个返回值应该是一个状态码,表示函数执行的结果。如果查询成功,应该返回状态码 `200`,表示成功。如果查询失败,应该返回一个错误码,表示失败的原因。根据你的代码逻辑,如果查询失败,应该返回状态码 `0`,而不是 `200`。同时,你应该在返回值中返回错误信息,便于调试和处理错误。例如:
```
func GetReportList(db *sql.DB, data *model.ReportReq) (int, string, string, string, string, string, string, string, string, string, string, string, string) {
var Report_Num, Report_Name, Hospital_Id, Hospital_Name, User_Id, Name, Gender, Age, Phone, Createtime_String, Report_Image string
sqlStr := "select report_num,report_name,hospital_id,hospital_name,user_id,name,gender,age,phone,createtime_string,report_image from report where name = ? limit 1"
err := db.QueryRow(sqlStr, data.Name).Scan(
&Report_Num,
&Report_Name,
&Hospital_Id,
&Hospital_Name,
&User_Id,
&Name,
&Gender,
&Age,
&Phone,
&Createtime_String,
&Report_Image)
if err != nil {
logger.Sugar.Info(err)
return 500, "查询失败", "", "", "", "", "", "", "", "", "", "", ""
}
return 200, "查询成功", Report_Num, Report_Name, Hospital_Id, Hospital_Name, User_Id, Name, Gender, Age, Phone, Createtime_String, Report_Image
}
```
这样,在函数调用处,你可以根据返回的状态码来判断函数执行的结果,例如:
```
status, message, reportNum, reportName, hospitalId, hospitalName, userId, name, gender, age, phone, createTime, reportImage := GetReportList(db, data)
if status == 200 {
// 处理查询成功的情况
} else {
// 处理查询失败的情况
fmt.Println(message)
}
```
相关推荐
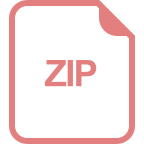
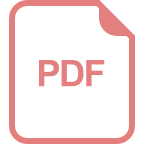
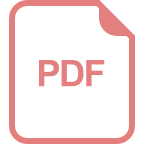














