用系统调用exec类函数对上述程序进行修改,实现父进程等待子进程运行,子进程执行新的任务,例如查看当前进程等等。
时间: 2024-12-16 14:22:37 浏览: 7
在C语言中,你可以使用`exec*`系列函数(如`execl`, `execlp`, `execv`, `execvp`, `execve`等)替换原本的`fork`+`write`+`close`+`wait`流程,以便在子进程中执行全新的任务。这里,我们使用`execl`函数,它允许你启动一个新的程序并替换当前进程。让我们假设你想让子进程执行`ps -ef`命令来查看当前进程列表:
首先,我们需要修改程序结构,将写入文件的部分删除,并在子进程部分使用`execl`调用一个新的shell,传入我们要执行的命令作为参数。父进程则直接退出,因为子进程接管了。
```c
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <sys/wait.h>
void run_command(char* cmd) {
execvp(cmd, cmd); // 执行新的命令
perror("Failed to execute command");
exit(1);
}
int main(int argc, char *argv[]) {
pid_t pid = fork();
if (pid == 0) { // 子进程
if (strcmp(argv[0], "./child") == 0) { // 判断是否来自子进程
run_command("/bin/bash"); // 进一步执行bash shell
} else { // 如果是原始进程,说明是测试示例,不做特殊处理
printf("This is the parent process.\n");
}
exit(0); // 子进程不再执行其他代码
} else if (pid > 0) { // 父进程
printf("Parent process waiting for child to finish.\n");
wait(NULL); // 等待子进程结束
printf("Child process finished.\n");
} else { // fork失败
perror("Failed to fork");
exit(1);
}
return 0;
}
```
在这个例子中,如果程序是从`./child`执行的(假设这是一个包含上述代码的独立程序),子进程将会启动一个bash shell,然后执行`ps -ef`命令。父进程会等待子进程完成后再继续执行。
**相关问题--:**
1. `execvp`和`execvppe`有何区别?
2. 使用`wait`函数前为什么要判断`pid == 0`?
3. 如果子进程执行的命令出错,如何捕获异常?
阅读全文
相关推荐
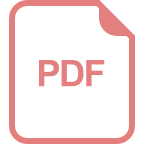
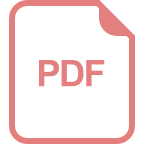
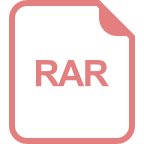















