android 自定义数字键盘
时间: 2023-07-28 09:59:55 浏览: 55
要自定义 Android 数字键盘,你可以使用自定义视图来替代默认的数字键盘。以下是一些步骤:
1.创建一个 XML 文件来定义你的自定义数字键盘的布局。例如,你可以在这个文件中添加按钮和其他视图,来表示数字键盘上的各个按键。
2.创建一个自定义视图类,该类继承自 View 或 ViewGroup,以便可以将你的 XML 布局文件加载到该视图中。
3.为你的自定义视图设置一些属性和方法,以便可以控制数字键盘的行为。例如,你可以添加一个方法来处理按键事件,或者添加一个属性来指定数字键盘的颜色和样式。
4.在你的应用程序中使用你的自定义数字键盘视图。这可以通过在 XML 布局文件中添加你的自定义视图,或者通过在代码中创建一个实例并将其添加到视图层次结构中来完成。
总之,自定义 Android 数字键盘需要你熟悉 Android 开发的基本知识,并且需要一些 Java 编程技巧。如果你不熟悉这些技术,可以参考一些 Android 开发教程或者请一个有经验的开发者来帮助你完成。
相关问题
Android 自定义软键盘
在 Android 中,自定义软键盘需要实现一个继承自 InputMethodService 的服务,这个服务会在用户打开软键盘时被调用。下面是一些步骤来创建自定义软键盘:
1. 创建一个新的 Android 项目,并在 AndroidManifest.xml 文件中声明一个新的服务:
```xml
<service
android:name=".CustomKeyboard"
android:label="Custom Keyboard"
android:permission="android.permission.BIND_INPUT_METHOD">
<meta-data
android:name="android.view.im"
android:resource="@xml/method" />
</service>
```
上面的代码声明了一个名为 CustomKeyboard 的服务,并将其与 android.view.im 绑定。在 res/xml 目录下创建一个名为 method.xml 的文件,用于指定 CustomKeyboard 的布局和行为:
```xml
<?xml version="1.0" encoding="utf-8"?>
<input-method xmlns:android="http://schemas.android.com/apk/res/android"
android:settingsActivity=".SettingsActivity"
android:imeSubtypeLocale="en_US"
android:imeSubtypeMode="keyboard" >
</input-method>
```
上面的代码指定了键盘的设置活动、语言环境和子类型模式。
2. 创建 CustomKeyboard 类,并继承 InputMethodService。在这个类中,你需要重写一些回调方法,例如 onCreateInputView()、onKeyDown() 和 onStartInputView() 等。这些方法将决定键盘的外观和行为。
```java
public class CustomKeyboard extends InputMethodService implements KeyboardView.OnKeyboardActionListener {
private KeyboardView keyboardView;
private Keyboard keyboard;
@Override
public View onCreateInputView() {
keyboardView = (KeyboardView) getLayoutInflater().inflate(R.layout.keyboard, null);
keyboard = new Keyboard(this, R.xml.qwerty);
keyboardView.setKeyboard(keyboard);
keyboardView.setOnKeyboardActionListener(this);
return keyboardView;
}
@Override
public void onStartInputView(EditorInfo info, boolean restarting) {
super.onStartInputView(info, restarting);
keyboardView.setPreviewEnabled(false);
}
@Override
public void onKey(int primaryCode, int[] keyCodes) {
InputConnection ic = getCurrentInputConnection();
switch (primaryCode) {
case Keyboard.KEYCODE_DELETE:
ic.deleteSurroundingText(1, 0);
break;
case Keyboard.KEYCODE_SHIFT:
// do something
break;
default:
char c = (char) primaryCode;
ic.commitText(String.valueOf(c), 1);
}
}
}
```
上面的代码创建了一个名为 CustomKeyboard 的类,并在 onCreateInputView() 方法中设置了键盘的布局和行为。在 onStartInputView() 方法中,我们禁用了键盘预览功能。在 onKey() 方法中,我们检查按下的键码并执行相应的操作。
3. 创建键盘布局。在 res/xml 目录下创建一个名为 qwerty.xml 的文件,用于指定键盘布局:
```xml
<?xml version="1.0" encoding="utf-8"?>
<Keyboard xmlns:android="http://schemas.android.com/apk/res/android"
android:keyWidth="10%p"
android:keyHeight="60dp"
android:horizontalGap="0px"
android:verticalGap="0px"
android:keyEdgeFlags="left">
<Row>
<Key android:keyLabel="q" android:keyEdgeFlags="left"/>
<Key android:keyLabel="w"/>
<Key android:keyLabel="e"/>
<Key android:keyLabel="r"/>
<Key android:keyLabel="t"/>
<Key android:keyLabel="y"/>
<Key android:keyLabel="u"/>
<Key android:keyLabel="i"/>
<Key android:keyLabel="o"/>
<Key android:keyLabel="p" android:keyEdgeFlags="right"/>
</Row>
<Row>
<Key android:keyLabel="a" android:keyEdgeFlags="left"/>
<Key android:keyLabel="s"/>
<Key android:keyLabel="d"/>
<Key android:keyLabel="f"/>
<Key android:keyLabel="g"/>
<Key android:keyLabel="h"/>
<Key android:keyLabel="j"/>
<Key android:keyLabel="k"/>
<Key android:keyLabel="l android:keyEdgeFlags="right"/>
</Row>
<Row>
<Key android:keyLabel="shift" android:horizontalGap="10%p" android:keyWidth="20%p" android:keyEdgeFlags="left" android:isModifier="true" android:isSticky="true"/>
<Key android:keyLabel="z"/>
<Key android:keyLabel=""/>
<Key android:keyLabel="c"/>
<Key android:keyLabel="v"/>
<Key android:keyLabel="b"/>
<Key android:keyLabel="n"/>
<Key android:keyLabel="m"/>
<Key android:keyLabel="delete" android:keyWidth="20%p" android:keyEdgeFlags="right" android:icon="@drawable/ic_delete"/>
</Row>
<Row>
<Key android:keyLabel="123" android:keyEdgeFlags="left" android:keyWidth="20%p"/>
<Key android:keyLabel=" " android:keyWidth="40%p"/>
<Key android:keyLabel="return" android:keyWidth="20%p" android:keyEdgeFlags="right"/>
</Row>
</Keyboard>
```
上面的代码指定了一个基本的 QWERTY 键盘布局,包含字母、数字和删除键。
4. 运行应用程序并测试自定义软键盘。在测试键盘时,你需要在 Android 设备的输入法设置中激活你的自定义键盘。
以上就是创建自定义软键盘的基本步骤,你可以根据需要修改键盘的布局和行为。
android写一个数字键盘
好的,下面是一个简单的 Android 数字键盘的实现步骤:
1. 创建一个新的 XML 布局文件,命名为 "keyboard.xml",并添加如下代码:
```
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:stretchColumns="*">
<TableRow>
<Button
android:id="@+id/button1"
android:text="1"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button2"
android:text="2"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button3"
android:text="3"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</TableRow>
<TableRow>
<Button
android:id="@+id/button4"
android:text="4"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button5"
android:text="5"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button6"
android:text="6"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</TableRow>
<TableRow>
<Button
android:id="@+id/button7"
android:text="7"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button8"
android:text="8"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button9"
android:text="9"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</TableRow>
<TableRow>
<Button
android:id="@+id/buttonClear"
android:text="Clear"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button0"
android:text="0"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/buttonDone"
android:text="Done"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</TableRow>
</TableLayout>
```
2. 在你的 Activity 中,创建一个键盘弹出的方法:
```
private void showKeyboard() {
// 加载布局文件并创建视图对象
LayoutInflater inflater = LayoutInflater.from(this);
View keyboardView = inflater.inflate(R.layout.keyboard, null);
// 创建一个 PopupWindow 对象
PopupWindow keyboardPopup = new PopupWindow(keyboardView,
ViewGroup.LayoutParams.WRAP_CONTENT,
ViewGroup.LayoutParams.WRAP_CONTENT);
// 显示 PopupWindow
keyboardPopup.showAtLocation(findViewById(R.id.editText1),
Gravity.BOTTOM, 0, 0);
// 设置按钮点击事件
Button button1 = (Button) keyboardView.findViewById(R.id.button1);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 处理按钮点击事件
editText.setText(editText.getText() + "1");
}
});
// 处理其他按钮的点击事件...
}
```
3. 在你的 Activity 中,创建一个点击事件监听器,当用户点击 EditText 控件时,弹出数字键盘:
```
editText.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showKeyboard();
}
});
```
4. 为“Clear”、“Done”按钮添加点击事件监听器,实现清空和隐藏数字键盘的功能:
```
Button buttonClear = (Button) keyboardView.findViewById(R.id.buttonClear);
buttonClear.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
editText.setText("");
}
});
Button buttonDone = (Button) keyboardView.findViewById(R.id.buttonDone);
buttonDone.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
keyboardPopup.dismiss();
}
});
```
这样就完成了一个简单的 Android 数字键盘的实现。当用户点击 EditText 控件时,弹出自定义的数字键盘,用户可以输入数字并进行清空和隐藏操作。
相关推荐
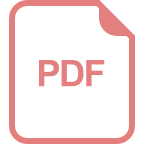











