#include <stdio.h> int main() { char str[100]; int count = 0; int i=0; while((str[i]=getchar())!='\n'){ i=i+1; } str[i]='\n'; for (int i = 0; str[i] != '\n'; i++) { if (str[i] == 'a' || str[i] == 'e' || str[i] == 'i' || str[i] == 'o' || str[i] == 'u') { count++; } } printf("%d", count); return 0; }如何优化
时间: 2023-10-25 14:09:56 浏览: 52
可以考虑以下几点来优化这段代码:
1. 字符串长度限制:可以使用fgets函数来读取输入的字符串,这样就可以限制输入字符串的长度,避免数组越界的错误。
2. 大小写不敏感:可以将输入的字符全部转换为小写字母,这样就可以避免大小写不一致的问题。
3. 元音字母判断:可以使用switch语句来替代多个if语句,使代码更加简洁。
下面是优化后的代码:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int count = 0;
fgets(str, 100, stdin);
for (int i = 0; str[i] != '\0'; i++) {
str[i] = tolower(str[i]); // 转换为小写字母
switch (str[i]) {
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
count++;
break;
}
}
printf("%d", count);
return 0;
}
```
优化后的代码使用了fgets函数来读取输入的字符串,并使用了头文件ctype.h中的函数tolower将输入字符串中的所有字符转换为小写字母。同时,使用了switch语句来判断元音字母,使代码更加简洁。
相关推荐
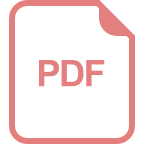
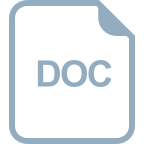
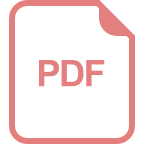















