#include<stdio.h> #include<time.h> #include<stdlib.h> #include<sys/ipc.h> #include<sys/shm.h> #include<string.h> #include <fcntl.h> #include <sys/types.h> #include<unistd.h> struct shared_msg{ char text[BUFSIZ]; int update; }; int num1,num2,i=0,count=0; static int shmid; char str1[100]={0},str2[100]={0},temp; struct shared_msg *msg=NULL; int main(){ //创建共享内存 shmid=shmget((key_t)1234,sizeof(struct shared_msg),IPC_CREAT); if(shmid<0){printf("错误,key 对应的共享内存已存在");exit(-1);} //映射共享内存 msg=(struct shared_msg *)shmat(shmid,0,0); if( msg < (struct shared_msg *)0){ printf("映射内存出错!\n"); exit(-2);} while(1){ while(msg->update==1||strcmp(msg->text,"end")==0){ //在屏幕上打印计算式 if(strcmp(msg->text,"end")==0){ printf("%s\n",msg->text); sprintf(msg->text,"over");return 0;} else {printf("%s\n",msg->text);} //获取第一个数据 if(msg->text[1]!='+'){ memcpy(str1,msg->text,2); str1[2]='\0';} else { memcpy(str1,msg->text,1); str1[1]='\0';} num1 = atoi(str1); //获取第二个数据 if(msg->text[1]!='+'){memcpy(str2,msg->text+3,2);} else {memcpy(str2,msg->text+2,2);} str2[2]='\0'; num2=atoi(str2); //把计算后的结果写入缓冲区 num1=num1+num2; sprintf(msg->text,"%d",num1); msg->update=0; } } return 0; }
时间: 2024-04-03 17:31:31 浏览: 13
这段代码是一个使用共享内存实现的简单计算器程序。它创建了一个共享内存区域,在进程间共享数据。其中 struct shared_msg 结构体定义了共享内存中的数据结构,包含一个字符串和一个整数,分别表示计算式和是否需要更新的标志。程序通过循环读取共享内存中的数据,当需要更新数据时,获取计算式中的两个数值,将它们相加后写入共享内存中,等待另一个进程读取。程序可以通过在计算式中输入 "end" 命令来结束运行。
相关问题
解释代码#include <sys/types.h> #include <sys/ipc.h> #include <sys/msg.h> #include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <string.h> #define MSGKEY 75 struct msgform { long mt
这段代码定义了一个消息队列,在 Linux 系统中,消息队列是一种进程间通信的方式。代码中包含了以下头文件:
- sys/types.h:定义了系统调用所使用的数据类型,例如 pid_t、size_t 等。
- sys/ipc.h:定义了用于进程间通信的 IPC(Inter-Process Communication)相关函数和数据结构,例如消息队列、信号量、共享内存等。
- sys/msg.h:定义了消息队列相关的函数和数据结构,例如 msgget、msgsnd、msgrcv 等。
- stdio.h:定义了输入输出函数,例如 printf、scanf 等。
- stdlib.h:定义了内存管理函数,例如 malloc、free 等。
- unistd.h:定义了一些 UNIX 标准的函数和符号常量,例如 sleep、fork、getpid 等。
- string.h:定义了一些字符串处理函数,例如 memcpy、memset 等。
在代码中,使用了宏定义 MSGKEY 定义了消息队列的键值。结构体 msgform 定义了消息的格式,包含了一个长整型变量 mt 和一个字符数组 mtext。后面的代码中使用了 msgget 函数获取消息队列的标识符,使用了 msgsnd 函数发送消息,使用了 msgrcv 函数接收消息。
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> #include <sys/wait.h> #include <sys/ipc.h> #include <sys/shm.h> #include <string.h> #define SIZE 128 int main(int argc,char *argv[]) { key_t key = ftok(".",'s'); if(key < 0) { perror("ftok"); return -1; } int shmid = shmget(key,SIZE,IPC_CREAT | 0777); if(shmid < 0) { perror("shmget"); return -1; } printf("shmid:%d\n",shmid); char *p = shmat(shmid,NULL,0); if((char *)-1 == p) { perror("shmat"); return -1; } while(1) { puts(p); sleep(1); if(strncmp(p,"quit",4) == 0) break; } if(-1 == shmdt(p)) { perror("shmdt"); exit(-1); } if(-1 == shmctl(shmid,IPC_RMID,NULL)) { perror("shmctl"); exit(-1); } return 0; }修改代码,使其正确输出读入端的代码一次
这里是修改后的代码,可以正确输出读入端的代码一次:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <sys/wait.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#define SIZE 128
int main(int argc, char *argv[]) {
key_t key = ftok(".", 's');
if (key < 0) {
perror("ftok");
return -1;
}
int shmid = shmget(key, SIZE, IPC_CREAT | 0777);
if (shmid < 0) {
perror("shmget");
return -1;
}
printf("shmid:%d\n", shmid);
char *p = shmat(shmid, NULL, 0);
if ((char *)-1 == p) {
perror("shmat");
return -1;
}
char buf[SIZE];
while (1) {
memset(buf, 0, SIZE);
fgets(buf, SIZE, stdin);
strncpy(p, buf, strlen(buf));
if (strncmp(p, "quit", 4) == 0)
break;
}
if (-1 == shmdt(p)) {
perror("shmdt");
exit(-1);
}
if (-1 == shmctl(shmid, IPC_RMID, NULL)) {
perror("shmctl");
exit(-1);
}
return 0;
}
```
相关推荐
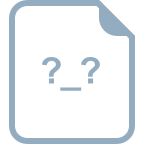
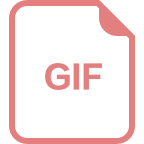
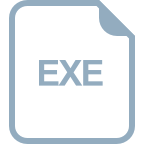














