此题和接下来的几道题要求你设计并实现一个Book类,你可以设想这是图书馆软件系统的一部分。Book类应包含表示ISBN号、书名、作者和版权日期以及表示是否已经借出的成员。创建能返回这些成员的值的函数,以及借书和还书的函数。对于输人Book对象的数据进行简单的有效性检查,例如,只接受n-n-n-x形式的ISBN号,其中n是一个整数,x是一个数字或一个字母。为 Book类添加运算符。添加==运算符,用于检查两本书的ISBN。ISBN号是否不等。定义<<,分行输出书名、作者和 ISBN号。为Book类创建一个名为Genre的枚举类型,用以区分书籍的类型:小说(fiction)、非小说类文学作品nonfiction)、期刊(periodical)、传记(biography)、儿童读物( children)。为每本书赋予一个Genre值,适当修改 Book的构造函数和成员函数。多文件程序,按文件给出代码
时间: 2024-02-13 11:59:47 浏览: 63
book.h文件:
```c++
#ifndef BOOK_H
#define BOOK_H
#include <iostream>
#include <string>
enum class Genre { fiction, nonfiction, periodical, biography, children };
class Book {
public:
Book(std::string isbn, std::string title, std::string author, std::string date, Genre genre, bool checked_out = false);
// 成员函数
std::string get_isbn() const { return isbn; }
std::string get_title() const { return title; }
std::string get_author() const { return author; }
std::string get_date() const { return date; }
bool is_checked_out() const { return checked_out; }
Genre get_genre() const { return genre; }
void check_out() { checked_out = true; }
void check_in() { checked_out = false; }
// 运算符重载
bool operator==(const Book& other) const;
bool operator!=(const Book& other) const { return !(*this == other); }
friend std::ostream& operator<<(std::ostream& os, const Book& book);
private:
std::string isbn;
std::string title;
std::string author;
std::string date;
Genre genre;
bool checked_out;
};
#endif // BOOK_H
```
book.cpp文件:
```c++
#include "book.h"
Book::Book(std::string isbn, std::string title, std::string author, std::string date, Genre genre, bool checked_out)
: isbn(isbn), title(title), author(author), date(date), genre(genre), checked_out(checked_out) {}
bool Book::operator==(const Book& other) const {
return isbn == other.isbn;
}
std::ostream& operator<<(std::ostream& os, const Book& book) {
os << book.title << "\n" << book.author << "\n" << book.isbn << "\n";
return os;
}
```
main.cpp文件:
```c++
#include "book.h"
#include <iostream>
using namespace std;
int main() {
Book book1("1-2-3-x", "C++ Primer", "Stanley B. Lippman", "2012/8/26", Genre::nonfiction);
Book book2("4-5-6-y", "The Catcher in the Rye", "J. D. Salinger", "1951/7/16", Genre::fiction);
// 测试成员函数
cout << book1.get_isbn() << endl;
cout << book1.get_title() << endl;
cout << book1.get_author() << endl;
cout << book1.get_date() << endl;
cout << book1.is_checked_out() << endl;
cout << static_cast<int>(book1.get_genre()) << endl;
book1.check_out();
cout << book1.is_checked_out() << endl;
book1.check_in();
cout << book1.is_checked_out() << endl;
// 测试运算符重载
cout << (book1 == book2) << endl;
cout << (book1 != book2) << endl;
cout << book1;
cout << book2;
return 0;
}
```
阅读全文
相关推荐
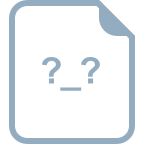
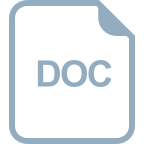
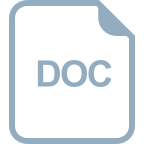


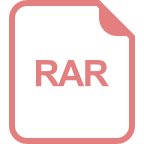
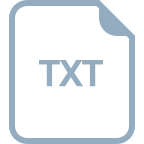
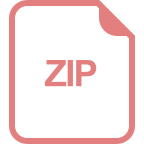
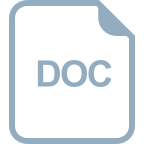
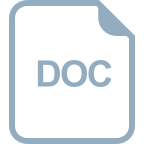
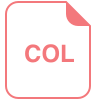
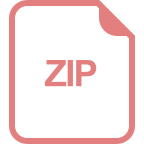
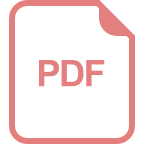
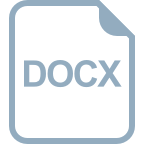
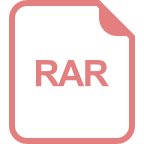
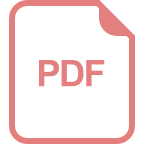