此题和接下来的几道题要求你设计并实现一个Book类,你可以设想这是图书馆软件系统的一部分。Book类应包含表示ISBN号、书名、作者和版权日期以及表示是否已经借出的成员。创建能返回这些成员的值的函数,以及借书和还书的函数。对于输人Book对象的数据进行简单的有效性检查,例如,只接受n-n-n-x形式的ISBN号,其中n是一个整数,是一个数字或一个字母。请用C++编写,
时间: 2024-02-15 11:04:01 浏览: 221
下面是一个基本的Book类的实现,包含ISBN号、书名、作者、版权日期和是否已经借出的成员,以及能返回这些成员的值的函数,以及借书和还书的函数。在构造函数中对ISBN号进行了简单的有效性检查。
```cpp
#include <iostream>
#include <string>
using namespace std;
class Book {
private:
string isbn; // ISBN号
string name; // 书名
string author; // 作者
string date; // 版权日期
bool borrowed; // 是否已经借出
public:
// 构造函数
Book(string i, string n, string a, string d): isbn(i), name(n), author(a), date(d), borrowed(false) {
// 简单的有效性检查
if (i.length() != 9 || i[1] != '-' || i[5] != '-' || i[7] != '-') {
cout << "ISBN号格式不正确!" << endl;
isbn = "";
}
}
// 返回ISBN号
string getIsbn() const {
return isbn;
}
// 返回书名
string getName() const {
return name;
}
// 返回作者
string getAuthor() const {
return author;
}
// 返回版权日期
string getDate() const {
return date;
}
// 返回是否已经借出
bool isBorrowed() const {
return borrowed;
}
// 借书
void borrow() {
if (borrowed) {
cout << "书已经被借出!" << endl;
} else {
borrowed = true;
}
}
// 还书
void giveBack() {
borrowed = false;
}
};
```
可以通过下面的代码进行测试:
```cpp
int main() {
Book book("1-23-456-7", "C++ Primer", "Lippman", "2020-01-01");
cout << "ISBN号:" << book.getIsbn() << endl;
cout << "书名:" << book.getName() << endl;
cout << "作者:" << book.getAuthor() << endl;
cout << "版权日期:" << book.getDate() << endl;
cout << "是否已经借出:" << book.isBorrowed() << endl;
book.borrow();
cout << "是否已经借出:" << book.isBorrowed() << endl;
book.giveBack();
cout << "是否已经借出:" << book.isBorrowed() << endl;
return 0;
}
```
阅读全文
相关推荐
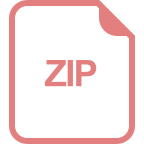
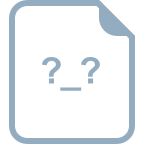

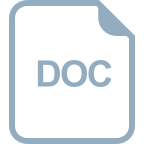
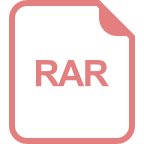
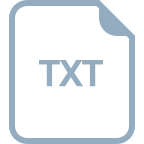
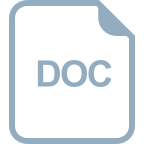
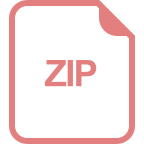
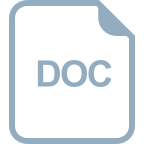
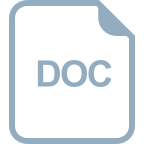
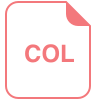
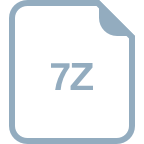
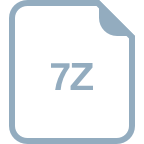
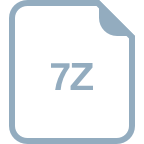
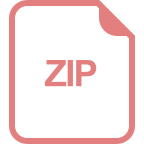
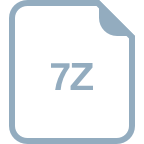
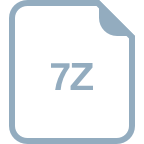