写基于TensorFlow Lite花卉识别的代码
时间: 2024-05-01 17:22:35 浏览: 11
首先,需要明确一下,在 TensorFlow Lite 中,图像分类(Image Classification)是一个已经训练好的模型,我们只需要将其下载到本地,然后载入到 TensorFlow Lite Interpreter 中即可使用。因此,本文将介绍如何使用 TensorFlow Lite 进行图像分类,而不是如何训练一个花卉识别模型。
以下是基于 TensorFlow Lite 进行花卉识别的代码示例:
```python
import tensorflow as tf
import numpy as np
from PIL import Image
# 下载并载入 TensorFlow Lite 模型
model_path = "path/to/tflite/model"
interpreter = tf.lite.Interpreter(model_path=model_path)
interpreter.allocate_tensors()
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
# 载入测试图片
image_path = "path/to/image"
image = Image.open(image_path)
image = image.resize((224, 224)) # 将图片大小调整为模型输入的大小
image = np.array(image)
image = np.expand_dims(image, axis=0) # 增加一个 batch 维度
# 输入图片到模型中进行预测
interpreter.set_tensor(input_details[0]['index'], image)
interpreter.invoke()
# 获取模型的输出结果
output = interpreter.get_tensor(output_details[0]['index'])
output = np.squeeze(output) # 去掉 batch 维度
predicted_label = np.argmax(output) # 获取最大概率的类别
# 打印预测结果
print("Predicted label:", predicted_label)
```
其中,需要注意以下几点:
- `model_path` 是 TensorFlow Lite 模型文件的路径。
- `input_details` 和 `output_details` 分别是模型的输入和输出详情,它们包含了模型输入和输出的形状、数据类型、名称等信息。
- `image_path` 是测试图片的路径,需要将其加载为 NumPy 数组,并将其大小调整为模型输入的大小(本例中为 224x224)。
- `interpreter.set_tensor` 将测试图片输入到模型中进行预测,其中 `input_details[0]['index']` 是模型输入张量的索引。
- `interpreter.get_tensor` 获取模型的输出结果,其中 `output_details[0]['index']` 是模型输出张量的索引。
- `np.argmax` 获取最大概率的类别。
以上就是基于 TensorFlow Lite 进行花卉识别的代码示例。需要注意的是,本例中使用的是已经训练好的模型,如果需要训练自己的花卉识别模型,请参考 TensorFlow 官方文档。
相关推荐
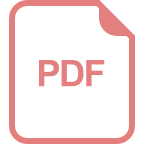














