可以帮我写一个Android实现两个数相乘后跳转到结果并能返回上一个页面的代码吗
时间: 2023-06-05 19:07:28 浏览: 48
当然可以,以下是一个简单的实现:
在xml文件中创建两个EditText,一个Button和一个TextView,分别用来输入两个数、执行乘法操作和显示结果。
```xml
<EditText
android:id="@+id/et_num1"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<EditText
android:id="@+id/et_num2"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/btn_calculate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Calculate"/>
<TextView
android:id="@+id/tv_result"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
```
在Java代码中获取EditText的值,执行乘法操作并将结果显示在TextView中。通过Intent将结果传递给下一个Activity,点击返回按钮可以返回上一个页面。
```java
// 获取控件对象
EditText etNum1 = findViewById(R.id.et_num1);
EditText etNum2 = findViewById(R.id.et_num2);
Button btnCalculate = findViewById(R.id.btn_calculate);
TextView tvResult = findViewById(R.id.tv_result);
// 设置计算按钮点击事件
btnCalculate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 获取两个数的值
int num1 = Integer.parseInt(etNum1.getText().toString());
int num2 = Integer.parseInt(etNum2.getText().toString());
// 计算结果
int result = num1 * num2;
// 将结果显示在TextView中
tvResult.setText(String.valueOf(result));
// 跳转到下一个页面
Intent intent = new Intent(MainActivity.this, ResultActivity.class);
intent.putExtra("result", result);
startActivity(intent);
}
});
// 在下一个页面通过Intent获取结果并显示
Intent intent = getIntent();
int result = intent.getIntExtra("result", 0);
TextView tvResult = findViewById(R.id.tv_result);
tvResult.setText(String.valueOf(result));
// 设置返回按钮点击事件
findViewById(R.id.btn_back).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 返回上一个页面
finish();
}
});
```
相关推荐
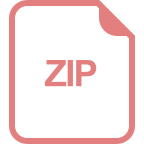














