用mapreduce实现二分法的代码
时间: 2023-06-14 08:05:15 浏览: 66
二分法是一种常见的查找算法,可以用 MapReduce 实现。下面是一个用 MapReduce 实现二分法的示例代码:
Mapper 阶段:
```java
public class BinarySearchMapper extends Mapper<LongWritable, Text, Text, Text> {
private int target;
@Override
protected void setup(Context context) throws IOException, InterruptedException {
// 从配置中获取目标数
target = context.getConfiguration().getInt("target", -1);
}
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// 将输入的数据转换成整数
int num = Integer.parseInt(value.toString());
// 二分查找
int left = 0, right = num - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (mid == target) {
context.write(new Text("result"), new Text(String.valueOf(mid)));
break;
} else if (mid < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
}
}
```
Reducer 阶段:
```java
public class BinarySearchReducer extends Reducer<Text, Text, Text, Text> {
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
for (Text value : values) {
context.write(key, value);
}
}
}
```
Driver 阶段:
```java
public class BinarySearchDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
conf.setInt("target", 50); // 设置目标数
Job job = Job.getInstance(conf, "Binary Search");
job.setJarByClass(BinarySearchDriver.class);
job.setMapperClass(BinarySearchMapper.class);
job.setReducerClass(BinarySearchReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上代码仅供参考,实际使用时还需要根据具体情况进行修改。
相关推荐
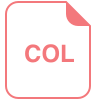
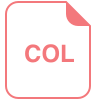
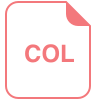
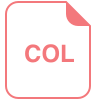
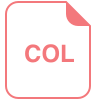









