用mapreduce实现倒排索引
时间: 2023-12-26 17:27:24 浏览: 59
倒排索引是一种常用的文本检索技术,可以快速找到包含某个词语的文档。下面是使用MapReduce实现倒排索引的示例代码:
首先,我们需要定义Mapper类,将输入的文档进行分词,并将每个词语作为key,文档ID作为value输出。
```java
public class InvertedIndexMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text word = new Text();
private Text docId = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] parts = line.split("\t"); // 假设每行的格式为:文档ID\t文本内容
if (parts.length == 2) {
String docId = parts[0];
String text = parts[1];
// 对文本进行分词
String[] words = text.split(" ");
// 输出每个词语和对应的文档ID
for (String word : words) {
this.word.set(word);
this.docId.set(docId);
context.write(this.word, this.docId);
}
}
}
}
```
接下来,我们需要定义Reducer类,将相同词语的文档ID进行合并,并输出倒排索引。
```java
public class InvertedIndexReducer extends Reducer<Text, Text, Text, Text> {
private Text result = new Text();
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
StringBuilder sb = new StringBuilder();
// 将相同词语的文档ID进行合并
for (Text value : values) {
sb.append(value.toString()).append(",");
}
this.result.set(sb.toString());
context.write(key, this.result);
}
}
```
最后,我们需要在主函数中配置和运行MapReduce作业。
```java
public class InvertedIndexJob {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Inverted Index");
job.setJarByClass(InvertedIndexJob.class);
job.setMapperClass(InvertedIndexMapper.class);
job.setReducerClass(InvertedIndexReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上代码实现了一个简单的倒排索引的MapReduce作业。你可以将输入的文档放在HDFS上,并将输入路径和输出路径作为命令行参数传递给作业。
相关推荐
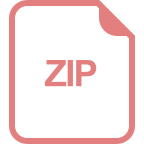
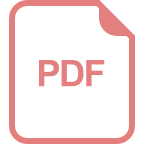














