用C语言问题描述:以一个m*n的长方阵表示迷宫,0和1分别表示迷宫中的通路和障碍。设计一个程序,对任意设定的迷宫,求出一条从入口到出口的通路,或得出没有通路的结论。
时间: 2023-09-17 20:06:38 浏览: 54
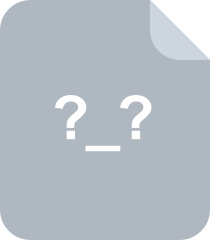
以一个mXn的长方阵表示迷宫, 0和1分别表示迷宫中的通路和障碍 设 计一个程序,对任意设定的迷宫,求出一条从入口到出口的通路
以下是用C语言描述的迷宫求解算法:
1. 定义一个结构体来表示迷宫中的一个位置,包括它在迷宫中的行列坐标和它的状态(是否为障碍)。
```c
struct point {
int x;
int y;
int status;
};
```
2. 定义一个栈来保存迷宫中的路径,即已经走过的位置。
```c
struct stack {
struct point data[MAXSIZE];
int top;
};
```
其中,MAXSIZE代表栈的最大容量,top表示栈顶元素的下标。
3. 定义一个二维数组来表示迷宫,0表示通路,1表示障碍。
```c
int maze[MAX_ROWS][MAX_COLS];
```
其中,MAX_ROWS和MAX_COLS分别表示迷宫的行数和列数。
4. 实现一个函数来读取迷宫的数据,将迷宫保存到二维数组中。
```c
void read_maze(int maze[][MAX_COLS], int rows, int cols) {
// 读取迷宫数据,将数据保存到maze数组中
}
```
5. 实现一个函数来判断一个位置是否为合法位置,即它在迷宫范围内且未被访问过且不是障碍。
```c
int is_valid(struct point p, int maze[][MAX_COLS]) {
if (p.x < 0 || p.x >= MAX_ROWS || p.y < 0 || p.y >= MAX_COLS || maze[p.x][p.y] == 1) {
return 0;
}
return 1;
}
```
6. 实现一个函数来判断是否到达了迷宫的出口。
```c
int is_exit(struct point p, int rows, int cols) {
if (p.x == rows-1 && p.y == cols-1) {
return 1;
}
return 0;
}
```
7. 实现一个函数来搜索迷宫中的路径,使用深度优先搜索算法。
```c
int dfs(int maze[][MAX_COLS], int rows, int cols) {
struct stack s;
struct point start = {0, 0, 0};
struct point next, cur;
s.top = -1;
s.data[++s.top] = start;
maze[0][0] = 1;
while (s.top != -1) {
cur = s.data[s.top];
if (is_exit(cur, rows, cols)) {
return 1;
}
next.x = cur.x + 1;
next.y = cur.y;
if (is_valid(next, maze)) {
s.data[++s.top] = next;
maze[next.x][next.y] = 1;
continue;
}
next.x = cur.x - 1;
next.y = cur.y;
if (is_valid(next, maze)) {
s.data[++s.top] = next;
maze[next.x][next.y] = 1;
continue;
}
next.x = cur.x;
next.y = cur.y + 1;
if (is_valid(next, maze)) {
s.data[++s.top] = next;
maze[next.x][next.y] = 1;
continue;
}
next.x = cur.x;
next.y = cur.y - 1;
if (is_valid(next, maze)) {
s.data[++s.top] = next;
maze[next.x][next.y] = 1;
continue;
}
--s.top;
}
return 0;
}
```
其中,使用栈来保存已经走过的路径,每次搜索时,先取出栈顶元素,然后依次判断它的四个邻居是否为合法位置,如果是,则将邻居加入到栈中,并标记为已经访问过。如果找到了出口,则返回1,否则返回0。
8. 在主函数中调用上述函数来求解迷宫路径。
```c
int main() {
int maze[MAX_ROWS][MAX_COLS];
int rows, cols;
// 读取迷宫数据,获取迷宫的行数和列数
if (dfs(maze, rows, cols)) {
printf("找到了迷宫的出口\n");
} else {
printf("没有找到迷宫的出口\n");
}
return 0;
}
```
阅读全文
相关推荐
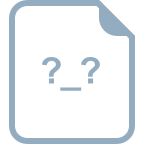








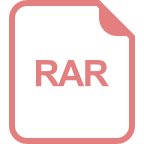
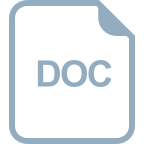
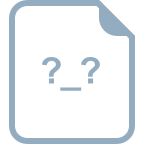