vue将docx文档转换为html
时间: 2024-02-23 12:01:17 浏览: 31
在Vue中将docx文档转换为HTML需要使用第三方库来实现。以下是一个使用JSZip和JSXlsx库来将docx转换为HTML的示例代码:
```javascript
import JSZip from 'jszip';
import JSXlsx from 'xlsx';
// 读取docx文件
const readFile = file => {
return new Promise((resolve, reject) => {
const reader = new FileReader();
reader.onload = () => resolve(reader.result);
reader.onerror = reject;
reader.readAsArrayBuffer(file);
});
};
// 将docx文件转换为HTML
const convertToHtml = async file => {
const content = await readFile(file);
const zip = await JSZip.loadAsync(content);
const docContent = await zip.file('word/document.xml').async('string');
const workbook = JSXlsx.read(docContent, { type: 'string' });
const sheet = workbook.Sheets[workbook.SheetNames[0]];
const html = JSXlsx.utils.sheet_to_html(sheet);
return html;
};
// 在Vue组件中使用
<template>
<div v-html="html"></div>
</template>
<script>
export default {
data() {
return {
html: ''
};
},
methods: {
async handleFileChange(event) {
const file = event.target.files[0];
const html = await convertToHtml(file);
this.html = html;
}
}
};
</script>
```
这个示例代码中,我们使用JSZip库读取docx文件,然后使用JSXlsx库将docx文件中的XML内容解析为一个xlsx工作簿对象。接着,我们将工作簿中的第一个sheet转换为HTML,并将HTML代码渲染到Vue组件中。如果你需要更复杂的处理流程,可以根据需要修改代码。
相关推荐
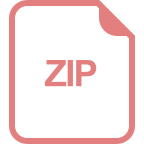
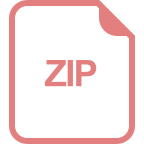
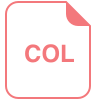
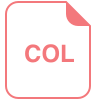
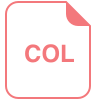
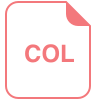
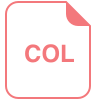









