基于easyx的贪吃蛇游戏C++
时间: 2023-12-30 13:04:03 浏览: 119
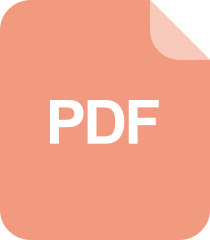
基于easyx的C++实现贪吃蛇

以下是基于easyx的贪吃蛇游戏C++的代码示例:
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
#include <stdlib.h>
#define WIDTH 800
#define HEIGHT 600
#define SIZE 20
struct Snake {
int x, y;
struct Snake *next;
};
struct Food {
int x, y;
};
int score = 0;
int high_score = 0;
int direction = VK_RIGHT;
struct Snake *head, *tail;
struct Food food;
void init();
void draw();
void update();
void generate_food();
bool check_collision(int x, int y);
void game_over();
void save_score();
void restart_game();
int main() {
init();
while (true) {
draw();
update();
Sleep(100);
}
return 0;
}
void init() {
initgraph(WIDTH, HEIGHT);
setbkcolor(BLACK);
setcolor(WHITE);
settextstyle(20, 0, _T("Consolas"));
head = new Snake{WIDTH / 2, HEIGHT / 2, NULL};
tail = head;
generate_food();
}
void draw() {
cleardevice();
// 画蛇
struct Snake *p = head;
while (p != NULL) {
fillrectangle(p->x, p->y, p->x + SIZE, p->y + SIZE);
p = p->next;
}
// 画食物
setfillcolor(RED);
fillcircle(food.x + SIZE / 2, food.y + SIZE / 2, SIZE / 2);
// 画分数
TCHAR str[20];
_stprintf_s(str, _T("Score: %d"), score);
outtextxy(10, 10, str);
_stprintf_s(str, _T("High Score: %d"), high_score);
outtextxy(10, 40, str);
}
void update() {
// 移动蛇头
int x = head->x, y = head->y;
switch (direction) {
case VK_LEFT:
x -= SIZE;
break;
case VK_RIGHT:
x += SIZE;
break;
case VK_UP:
y -= SIZE;
break;
case VK_DOWN:
y += SIZE;
break;
}
// 检查是否吃到食物
if (x == food.x && y == food.y) {
score++;
if (score > high_score) {
high_score = score;
}
generate_food();
} else {
// 移动蛇尾
tail->x = x;
tail->y = y;
head->next = tail;
head = tail;
tail = tail->next;
}
// 检查是否撞到墙或自己
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT || check_collision(x, y)) {
game_over();
}
}
void generate_food() {
srand((unsigned)time(NULL));
do {
food.x = rand() % (WIDTH / SIZE) * SIZE;
food.y = rand() % (HEIGHT / SIZE) * SIZE;
} while (check_collision(food.x, food.y));
}
bool check_collision(int x, int y) {
struct Snake *p = tail;
while (p != head) {
if (p->x == x && p->y == y) {
return true;
}
p = p->next;
}
return false;
}
void game_over() {
save_score();
TCHAR str[20];
_stprintf_s(str, _T("Game Over! Score: %d"), score);
MessageBox(NULL, str, _T("Game Over"), MB_OK);
restart_game();
}
void save_score() {
FILE *fp;
fopen_s(&fp, "score.txt", "w");
fprintf_s(fp, "%d", high_score);
fclose(fp);
}
void restart_game() {
score = 0;
direction = VK_RIGHT;
struct Snake *p = tail->next;
while (p != NULL) {
struct Snake *q = p;
p = p->next;
delete q;
}
head = new Snake{WIDTH / 2, HEIGHT / 2, NULL};
tail = head;
generate_food();
}
--相关问题--:
阅读全文
相关推荐
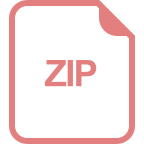

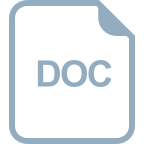
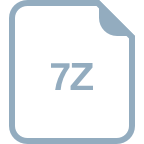
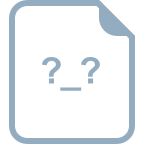
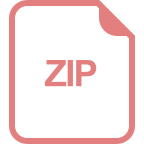
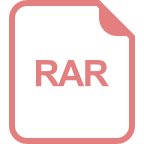
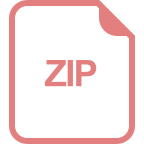
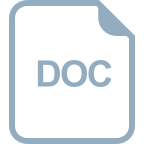
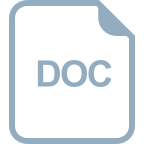
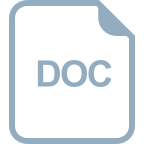
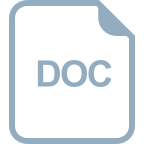
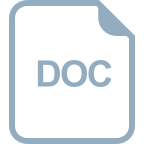
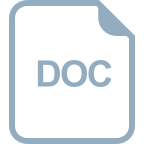

