一次性用c++写出一个学生管理系统
时间: 2023-04-08 22:03:01 浏览: 67
好的,我可以回答这个问题。一个基本的学生管理系统需要包括以下功能:添加学生信息、删除学生信息、修改学生信息、查询学生信息、显示所有学生信息等。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENT_NUM 100
struct Student {
char name[20];
int age;
char gender[10];
char major[20];
};
struct Student students[MAX_STUDENT_NUM];
int student_num = 0;
void add_student() {
if (student_num >= MAX_STUDENT_NUM) {
printf("The student list is full.\n");
return;
}
struct Student new_student;
printf("Please enter the student's name: ");
scanf("%s", new_student.name);
printf("Please enter the student's age: ");
scanf("%d", &new_student.age);
printf("Please enter the student's gender: ");
scanf("%s", new_student.gender);
printf("Please enter the student's major: ");
scanf("%s", new_student.major);
students[student_num++] = new_student;
printf("The student has been added successfully.\n");
}
void delete_student() {
if (student_num == 0) {
printf("The student list is empty.\n");
return;
}
char name[20];
printf("Please enter the name of the student you want to delete: ");
scanf("%s", name);
int index = -1;
for (int i = 0; i < student_num; i++) {
if (strcmp(students[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("The student is not found.\n");
return;
}
for (int i = index; i < student_num - 1; i++) {
students[i] = students[i + 1];
}
student_num--;
printf("The student has been deleted successfully.\n");
}
void modify_student() {
if (student_num == 0) {
printf("The student list is empty.\n");
return;
}
char name[20];
printf("Please enter the name of the student you want to modify: ");
scanf("%s", name);
int index = -1;
for (int i = 0; i < student_num; i++) {
if (strcmp(students[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("The student is not found.\n");
return;
}
printf("Please enter the new age of the student: ");
scanf("%d", &students[index].age);
printf("Please enter the new gender of the student: ");
scanf("%s", students[index].gender);
printf("Please enter the new major of the student: ");
scanf("%s", students[index].major);
printf("The student has been modified successfully.\n");
}
void query_student() {
if (student_num == 0) {
printf("The student list is empty.\n");
return;
}
char name[20];
printf("Please enter the name of the student you want to query: ");
scanf("%s", name);
int index = -1;
for (int i = 0; i < student_num; i++) {
if (strcmp(students[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("The student is not found.\n");
return;
}
printf("Name: %s\nAge: %d\nGender: %s\nMajor: %s\n", students[index].name, students[index].age, students[index].gender, students[index].major);
}
void show_all_students() {
if (student_num == 0) {
printf("The student list is empty.\n");
return;
}
printf("Name\tAge\tGender\tMajor\n");
for (int i = 0; i < student_num; i++) {
printf("%s\t%d\t%s\t%s\n", students[i].name, students[i].age, students[i].gender, students[i].major);
}
}
int main() {
while (1) {
printf("Please select an operation:\n");
printf("1. Add a student\n");
printf("2. Delete a student\n");
printf("3. Modify a student\n");
printf("4. Query a student\n");
printf("5. Show all students\n");
printf("6. Exit\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
add_student();
break;
case 2:
delete_student();
break;
case 3:
modify_student();
break;
case 4:
query_student();
break;
case 5:
show_all_students();
break;
case 6:
exit(0);
default:
printf("Invalid choice.\n");
break;
}
}
return 0;
}
```
阅读全文
相关推荐
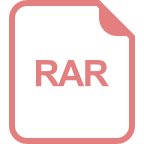
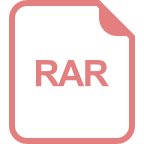
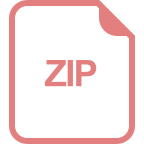
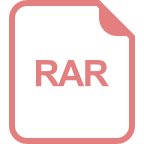
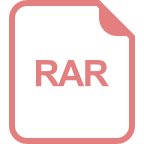
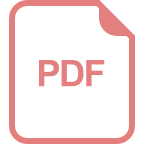
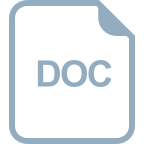
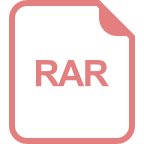
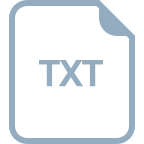
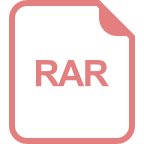
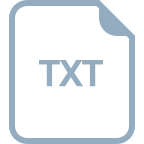
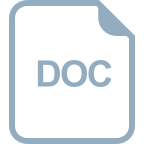
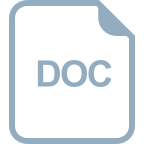
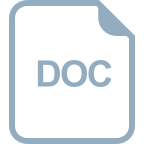
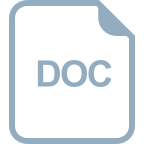