(二)实现获取字符串中的每个元素并输出+ (1)定义一个字符串,用你们的姓名和学号作为这个字符串的内容,如“张三112332245”;←(2)获取这个字符串的每个元素,并输出每个元素;+ (3)统计这个字符串的字符数量,并输出结果:“该字符串共包含x个字符”(4现 (三)找出指定列表中单词最短的一个。+(1列表a=[aaabbbbcccccc]+(2)函数实现
时间: 2024-03-08 08:47:22 浏览: 47
好的,我会逐步回答您的问题。
(二)获取字符串中的每个元素并输出:
1. 定义一个字符串:
```
str = "张三112332245"
```
2. 获取每个元素并输出:
```
for char in str:
print(char)
```
输出结果为:
```
张
三
1
1
2
3
3
2
2
4
5
```
3. 统计字符串的字符数量并输出:
```
count = len(str)
print("该字符串共包含{}个字符".format(count))
```
输出结果为:
```
该字符串共包含11个字符
```
(三)找出指定列表中单词最短的一个:
1. 定义列表:
```
a = ['aaabbbbcccccc']
```
2. 实现函数:
```
def shortest_word(words):
shortest = words[0]
for word in words:
if len(word) < len(shortest):
shortest = word
return shortest
```
3. 调用函数并输出结果:
```
shortest = shortest_word(a)
print("列表中单词最短的是: {}".format(shortest))
```
输出结果为:
```
列表中单词最短的是: aaabbbbcccccc
```
相关问题
使用c语言,将自己的学号与姓名拼音组成的字符串,换为ASCII码输出
在C语言中,你可以使用`printf`函数来打印出字符串的每个字符的ASCII码。下面是一个简单的C语言程序示例,它会读取学生的学号和姓名,并将每个字符转换为其对应的ASCII码并输出:
```c
#include <stdio.h>
int main() {
char studentID[100]; // 假设学号不超过99位
char name[100]; // 假设姓名拼音不超过99个字符
// 获取用户输入的学号和姓名
printf("请输入您的学号: ");
scanf("%99s", studentID); // 读取学号
printf("请输入您的姓名拼音: ");
scanf("%99s", name); // 读取姓名
// 输出学号和姓名每个字符的ASCII码
printf("学号的ASCII码为:\n");
for (int i = 0; studentID[i] != '\0'; i++) {
printf("%d ", (unsigned char)studentID[i]); // 使用(unsigned char)确保打印的是正数
}
printf("\n\n姓名的ASCII码为:\n");
for (int i = 0; name[i] != '\0'; i++) {
printf("%d ", (unsigned char)name[i]); // 使用(unsigned char)确保打印的是正数
}
printf("\n");
return 0;
}
```
这个程序首先定义了两个字符数组用于存储学号和姓名。通过`scanf`函数获取用户输入的学号和姓名。之后,使用两个`for`循环遍历这两个字符串,打印出每个字符的ASCII码值。注意,在`printf`中使用`(unsigned char)`将字符强制转换为无符号字符,以确保输出的是正数值的ASCII码。
用c语言编写定义嵌套结构体Student然后从终端上输入以下五个学生的学号姓名和出生日期并输出
在C语言中,可以创建一个嵌套结构体来表示学生信息,包括学号、姓名和出生日期。首先,我们需要定义一个结构体`Student`,接着定义包含`Student`的另一个结构体`PersonalInfo`,最后编写主函数来接受用户输入并打印。
```c
#include <stdio.h>
#include <string.h> // 需要用到字符串库
// 定义嵌套结构体
typedef struct PersonalInfo {
char name[50]; // 姓名
char birthDate[20]; // 出生日期,假设格式如"YYYY-MM-DD"
} PersonalInfo;
typedef struct Student {
int studentID; // 学号
PersonalInfo info; // 包含姓名和出生日期的信息
} Student;
void inputStudent(Student *student) {
printf("请输入学生信息(学号、姓名、出生日期):\n");
scanf("%d %s %s", &student->studentID, student->info.name, student->info.birthDate);
}
void printStudent(Student student) {
printf("学生信息:\n");
printf("学号: %d\n", student.studentID);
printf("姓名: %s\n", student.info.name);
printf("出生日期: %s\n", student.info.birthDate);
}
int main() {
Student students[5]; // 创建一个包含5个学生信息的数组
// 为每个学生输入信息
for (int i = 0; i < 5; i++) {
inputStudent(&students[i]);
}
// 打印所有学生的信息
for (int i = 0; i < 5; i++) {
printStudent(students[i]);
printf("\n-------------------------\n"); // 每个学生信息间添加分隔线
}
return 0;
}
```
在这个程序中,`inputStudent`函数用于获取用户输入,`printStudent`函数用于展示学生信息。在`main`函数中,我们创建了一个大小为5的`Student`数组,循环遍历输入和打印每个学生的数据。
阅读全文
相关推荐
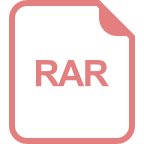















