tensor = np.array(iput[ep]).reshape(3, 3, 3) ValueError: cannot reshape array of size 9 into shape (3,3,3)
时间: 2024-05-28 09:14:20 浏览: 112
这个错误提示是因为你正在尝试将一个大小为9的数组重新形状为3x3x3的数组,这是不可能的。请检查你的代码并确保输入数据的大小与你尝试重塑的形状相匹配。你可以使用`np.shape()`函数来检查数组的大小和形状。如果你确信数据大小和形状是正确的,你可以尝试使用`np.reshape()`函数而不是`.reshape()`方法来重塑数组。
相关问题
tensor = np.array(iput[ep]).reshape(1, 3, 3) ValueError: cannot reshape array of size 3 into shape (1,3,3)
这个错误是因为你的 `input[ep]` 的大小为 3,而你尝试将其转换为一个大小为 (1, 3, 3) 的张量。这是不可能的,因为你只有 3 个元素,而在一个大小为 (1, 3, 3) 的张量中需要有 9 个元素。
你需要检查一下你的代码,确认你正在使用正确的输入数据,并且正在正确地处理和转换它们。如果你仍然无法解决问题,请提供更多的上下文或代码,以便我可以帮助你更好地解决问题。
# Transform a board(matrix) to a tensor def board2tensor(board, color, last_move, reshape_flag=True): # Current-Stone Layer cur = np.array(np.array(board) == color, dtype=np.int) # Enemy-Stone Layer e = np.array(np.array(board) == -color, dtype=np.int) # Last Step Layer l = np.zeros((board.shape[0], board.shape[1])) if last_move is not None: l[last_move[0]][last_move[1]] = 1 # Color Layer # flag = (1 if color == BLACK else 0) # c = flag * np.ones((board.shape[0], board.shape[1])) # Stack cur,e,c into tensor tensor = np.array([cur, e, l]) if reshape_flag: tensor = tensor.reshape(1, tensor.shape[0], tensor.shape[1], tensor.shape[2]) return tensor
这段代码是一个将棋盘(matrix)转换成张量(tensor)的函数。其中,参数board表示棋盘,color表示当前棋子的颜色,last_move表示上一次落子的位置。函数首先将棋盘中当前棋子的位置和对手棋子的位置分别转换成二值矩阵,然后将上一次落子的位置转换成只有一个位置值为1的矩阵。最后将这三个矩阵堆叠成一个张量,如果reshape_flag为True,则将其reshape成一个4维张量。
阅读全文
相关推荐








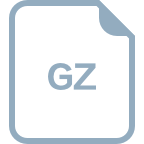
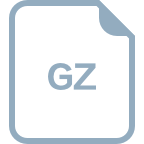







