kthread_run
时间: 2023-08-22 12:09:02 浏览: 133
kthread_run是Linux内核中的一个宏,它实际上是对kthread_create函数的一个封装。kthread_run的函数原型如下:
```
#define kthread_run(threadfn, data, namefmt, ...) \
kthread_create(threadfn, data, namefmt, ##__VA_ARGS__)
```
它的参数与kthread_create函数相同,只是省去了返回值。kthread_run函数会调用kthread_create函数创建一个内核线程,并立即启动它。与kthread_create不同的是,kthread_run会将内核线程的task_struct结构体中的state字段设置为TASK_RUNNING,表示线程已经处于运行状态。
kthread_run的作用与kthread_create类似,都是创建一个内核线程并运行它。不同之处在于kthread_run更加简单,不需要手动启动线程,也不需要手动设置线程状态。
相关问题
kthread_run实现10秒线程检测温度
kthread_run是Linux内核中用于创建内核线程的函数,它会创建一个新的内核线程,让它在后台运行,并且不会阻塞调用线程。如果你想要在内核线程中实现10秒线程检测温度,可以按照以下步骤:
1. 创建内核线程:使用kthread_run函数创建一个新的内核线程,将任务函数作为参数传递给它。
2. 编写任务函数:任务函数负责实现温度检测逻辑,可以使用Linux内核中已经提供的温度传感器驱动程序来读取温度值。
3. 设置定时器:使用定时器机制,例如使用timer_setup函数创建一个新的定时器,并将定时器回调函数设置为任务函数。
4. 启动定时器:在任务函数中启动定时器,让它在10秒后再次执行任务函数,并重新设置定时器。
5. 等待内核线程结束:使用kthread_stop函数等待内核线程执行完毕并结束。
下面是一个简单的代码示例:
```
#include <linux/kthread.h>
#include <linux/timer.h>
static struct task_struct *my_thread;
static struct timer_list my_timer;
void my_task_func(unsigned long data)
{
// 读取温度值并进行处理
// ...
// 重新设置定时器
mod_timer(&my_timer, jiffies + msecs_to_jiffies(10000));
}
int init_module(void)
{
// 创建内核线程
my_thread = kthread_run(my_task_func, NULL, "my_thread");
// 设置定时器
timer_setup(&my_timer, my_task_func, 0);
// 启动定时器
mod_timer(&my_timer, jiffies + msecs_to_jiffies(10000));
return 0;
}
void cleanup_module(void)
{
// 停止定时器
del_timer(&my_timer);
// 等待内核线程结束
kthread_stop(my_thread);
}
```
在这个示例中,my_task_func函数是任务函数,它会读取温度值并进行处理。my_timer是定时器对象,它会在10秒后再次执行任务函数。init_module函数是模块初始化函数,在其中创建内核线程,并设置和启动定时器。cleanup_module函数是模块清理函数,在其中停止定时器,并等待内核线程结束。
kthread_run实现10秒钟检测一次温度
首先,需要明确一下操作系统和处理器架构,因为不同的操作系统和处理器架构可能会有不同的实现细节。假设我们的操作系统是 Linux,并且使用 x86 架构的处理器。
在 Linux 中,可以使用 `/sys/class/thermal/thermal_zone0/temp` 文件读取 CPU 温度,其中 `thermal_zone0` 是一个虚拟的 thermal zone,表示 CPU 这个热区。该文件的格式为一个整数,表示当前温度以毫摄氏度为单位。
下面是一个使用 kthread_run 实现每 10 秒钟检测一次温度的示例代码:
```c
#include <linux/kernel.h>
#include <linux/kthread.h>
#include <linux/delay.h>
static int temperature = 0;
static int temp_thread(void *data)
{
while (!kthread_should_stop()) {
// 读取温度
int temp;
char buf[32];
struct file *file = filp_open("/sys/class/thermal/thermal_zone0/temp", O_RDONLY, 0);
if (IS_ERR(file)) {
printk(KERN_ERR "Failed to open thermal_zone0: %ld\n", PTR_ERR(file));
temp = -1;
} else {
ssize_t count = kernel_read(file, buf, sizeof(buf) - 1, 0);
if (count <= 0) {
printk(KERN_ERR "Failed to read thermal_zone0\n");
temp = -1;
} else {
buf[count] = '\0';
temp = simple_strtol(buf, NULL, 10) / 1000;
}
filp_close(file, NULL);
}
// 更新全局变量
temperature = temp;
// 暂停 10 秒钟
set_current_state(TASK_INTERRUPTIBLE);
schedule_timeout(HZ * 10);
}
return 0;
}
static struct task_struct *temp_task;
static int __init temp_init(void)
{
temp_task = kthread_run(temp_thread, NULL, "temp");
if (IS_ERR(temp_task)) {
printk(KERN_ERR "Failed to start temp thread: %ld\n", PTR_ERR(temp_task));
return PTR_ERR(temp_task);
}
return 0;
}
static void __exit temp_exit(void)
{
kthread_stop(temp_task);
}
module_init(temp_init);
module_exit(temp_exit);
```
在这个示例代码中,我们使用了一个全局变量 `temperature` 来保存当前温度。在 `temp_thread` 函数中,我们每 10 秒钟读取一次温度,并更新全局变量。在模块初始化时,我们启动一个 kernel 线程来执行 `temp_thread` 函数;在模块退出时,我们停止该线程。
阅读全文
相关推荐
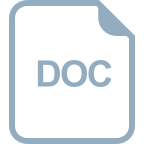
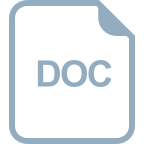
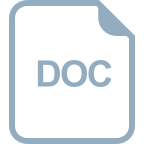





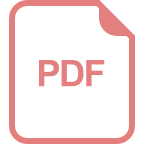
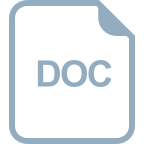
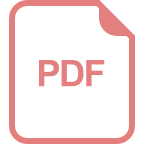





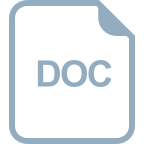