写一个爬虫: 1.网站地址:https://news.pdsu.edu.cn/xxyw.htm 2.获取底部分页页码 3.获取学校要闻中每页的所有新闻的标题、链接和发布日期 4.将标题、链接和发布日期存入excel
时间: 2024-12-17 19:43:19 浏览: 8
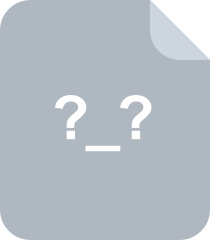
opencv-contrib-python-4.6.0.66-cp36-abi3-win-amd64.whl
要编写一个爬虫来抓取指定网站的信息并将其保存到Excel文件中,你可以使用Python的requests库来发送HTTP请求,BeautifulSoup库解析HTML内容,以及pandas库处理数据并导出到Excel。下面是一个简单的示例,假设我们使用了`lxml`库作为BeautifulSoup的一个替代版本:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 网站URL
url = "https://news.pdsu.edu.cn/xxyw.htm"
# Excel文件名
output_excel = 'news_data.xlsx'
def get_page_content(url):
# 发送GET请求
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 使用BeautifulSoup解析页面内容
soup = BeautifulSoup(response.text, 'lxml')
return soup
else:
print(f"Failed to fetch page with status {response.status_code}")
return None
def extract_news(soup):
news_list = []
# 查找新闻列表元素
news_elements = soup.find_all('div', class_='list-item')
for element in news_elements:
title = element.find('h2', class_='title').text.strip()
link = element.find('a')['href']
date = element.find('span', class_='date') or element.find('li', class_='time').text
news_list.append({'标题': title, '链接': link, '发布日期': date})
return news_list
def save_to_excel(data, filename):
df = pd.DataFrame(data)
df.to_excel(filename, index=False)
def main():
soup = get_page_content(url)
if soup is not None:
all_news = []
# 获取底部分页页码,这里假设每个底部分页链接都有数字
page_links = [link for link in soup.find_all('a') if str(link).isdigit()]
for i, link in enumerate(page_links):
next_url = f"{url}?page={i+1}"
news_page_soup = get_page_content(next_url)
if news_page_soup:
all_news.extend(extract_news(news_page_soup))
# 存储所有新闻数据到Excel
save_to_excel(all_news, output_excel)
print(f"数据已成功保存至{output_excel}")
if __name__ == "__main__":
main()
```
这个脚本会遍历每一页面的新闻,并将标题、链接和发布日期存入一个字典列表,然后将这个列表转换成DataFrame并写入Excel文件。注意,实际操作中你可能需要根据网站的具体结构调整CSS选择器。
阅读全文
相关推荐
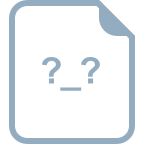
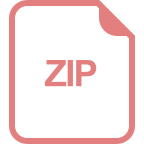



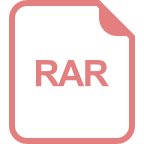
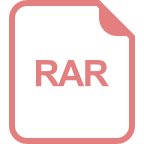
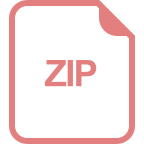
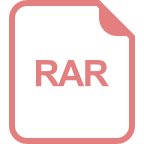
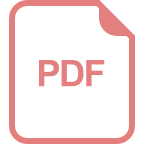
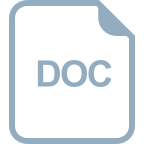
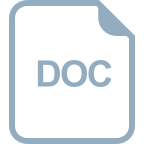

