写一个爬虫: 1.网站地址:https://news.pdsu.edu.cn/xxyw.htm 2.获取前三页底部分页页码 3.获取学校要闻中每页的所有新闻的标题、链接和发布日期 4.将标题、链接和发布日期存入excel
时间: 2024-12-18 14:37:31 浏览: 8
为了编写一个爬虫来完成这个任务,我们可以使用Python的requests库来发送HTTP请求,BeautifulSoup库来解析HTML内容,以及pandas库来处理Excel数据。下面是一个简化的步骤说明:
1. 首先安装必要的库:
```bash
pip install requests beautifulsoup4 pandas openpyxl
```
2. 创建一个Python脚本(例如`web_crawler.py`)并导入所需的模块:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
from openpyxl import Workbook
```
3. 定义函数来获取页面内容:
```python
def get_html(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
print(f"Failed to fetch {url}. Status code: {response.status_code}")
return None
def parse_news(html):
soup = BeautifulSoup(html, 'html.parser')
news_list = []
# 提取每页新闻的信息
# 这里需要分析网页结构找到正确的元素,假设新闻信息在'title', 'link', 和 'pub_date' 标签下
for item in soup.find_all('div', class_='news-item'):
title = item.find('h2').text
link = item.find('a')['href']
pub_date = item.find('span', class_='pub-date').text
news_list.append({'title': title, 'link': link, 'pub_date': pub_date})
return news_list
```
4. 获取前三页的页码和新闻:
```python
url_template = "https://news.pdsu.edu.cn/xxyw.htm?page={}"
total_pages = 3
page_numbers = list(range(1, total_pages + 1))
all_news = []
for page_num in page_numbers:
html = get_html(url_template.format(page_num))
if html:
parsed_news = parse_news(html)
all_news.extend(parsed_news)
# 获取总页数
last_page_number = max([int(link.split('=')[-1]) for link in set([link.split('/')[-1] for link in all_news if 'page=' in link])])
```
5. 将数据保存到Excel:
```python
# 创建一个空的Excel工作簿
wb = Workbook()
ws = wb.active
ws.title = "School News"
# 将数据写入Excel
for news in all_news:
ws.append(news.values())
# 保存文件
file_name = "news_data.xlsx"
wb.save(file_name)
print(f"Crawled data saved to {file_name}")
```
注意:上述代码示例是基于假设HTML结构的,实际操作中你需要查看目标网站的具体HTML结构来定位和提取所需的数据。由于网页结构可能会改变,你需要定期更新解析逻辑。
阅读全文
相关推荐
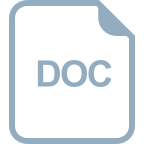
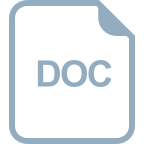
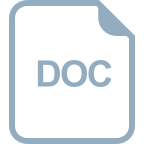



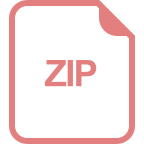
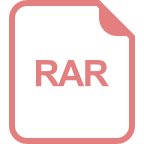
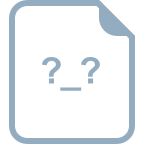
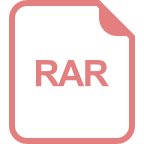
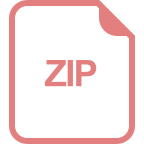
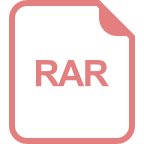
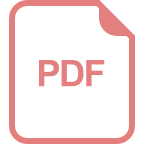
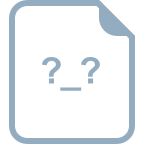
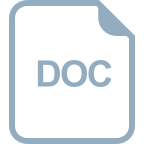


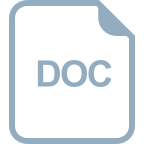