输入两个字符串,从第一个字符串中删除第二个字符串中的所有字符
时间: 2023-06-01 10:01:46 浏览: 120
### 回答1:
可以使用字符串的replace()方法,将第一个字符串中所有出现在第二个字符串中的字符替换为空字符串。代码示例:
```
str1 = input("请输入第一个字符串:")
str2 = input("请输入第二个字符串:")
for char in str2:
str1 = str1.replace(char, "")
print("删除后的字符串为:", str1)
```
### 回答2:
这个问题需要我们遵循以下步骤:
1. 首先确定两个字符串,分别为str1和str2;
2. 然后遍历str2字符串,循环每次取出一个字符;
3. 在遍历的过程中,每获取到一个字符,就在str1字符串中查找并删除该字符;
4. 最终输出删除后的str1字符串。
下面我们来具体解析一下这个过程。
首先,我们需要先确定两个字符串,这里我们就随便定一个str1为“abcdefg”,而str2为“abc”。
接下来,我们需要开始遍历str2字符串,代码如下:
```
for (int i = 0; i < str2.length(); i++) {
char ch = str2.charAt(i);
// 在str1中查找并删除该字符
}
```
在遍历的过程中,我们使用charAt()方法遍历str2字符串,并用一个字符变量ch来保存当前遍历到的字符。接下来,我们需要在str1字符串中查找并删除该字符,代码如下:
```
for (int i = 0; i < str2.length(); i++) {
char ch = str2.charAt(i);
int index = str1.indexOf(ch);
while (index != -1) {
str1 = str1.substring(0, index) + str1.substring(index + 1);
index = str1.indexOf(ch);
}
}
```
在代码中,我们使用indexOf()方法在str1字符串中查找字符ch的位置,若不存在则返回-1,否则返回该字符在字符串中的下标。接着,我们使用while循环不断删除该字符直到它不存在为止。具体来说,我们使用substring()方法将该字符从str1中删除,其中substring(0, index)表示取字符串str1中下标为0到index-1的子字符串,而substring(index + 1)则表示取字符串str1中下标为index+1到末尾的子字符串。
最后,我们输出删除后的str1字符串,代码如下:
```
System.out.println(str1);
```
综上所述,我们完成了从第一个字符串中删除第二个字符串中的所有字符的任务。
### 回答3:
这道题目可以使用两种方法来解决:暴力法和哈希表法。
暴力法的思路比较简单,就是从第一个字符串的第一个字符开始,逐一查找是否存在于第二个字符串中,如果存在则在第一个字符串中删除,继续查找下一个字符。这种方法的时间复杂度是O(n*m),其中n和m分别代表两个字符串的长度,因为需要对第一个字符串中每一个字符都进行查找和删除操作。
哈希表法则是通过先将第二个字符串中的每一个字符存储到一个哈希表中,然后再遍历第一个字符串中的每一个字符,判断其是否在哈希表中出现过,如果出现过则在第一个字符串中删除,继续查找下一个字符。这种方法的时间复杂度是O(n),因为只需要遍历一次第一个字符串。
下面是使用哈希表法的具体步骤:
1. 将第二个字符串中的每一个字符存储到一个哈希表中;
2. 遍历第一个字符串中的每一个字符,判断其是否在哈希表中出现过;
3. 如果出现过则在第一个字符串中删除该字符;
4. 继续查找下一个字符,重复以上步骤直到遍历完所有字符;
5. 返回最终结果。
需要注意的是,为了避免重复删除字符,可以使用一个指针来记录删除后的新字符串长度,然后在循环中更新指针位置,保证每次删除字符后指针位置可以正确更新。同时,在遍历时也需要从后往前遍历,否则会出现字符顺序错误的情况。
综上所述,使用哈希表法进行删除操作可以达到较高的时间复杂度效率和较好的删除效果。
相关推荐
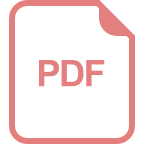
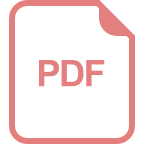














