以car.data中数据为样本,以Class Values为分类依据,利用样本的前80%数据建立分类模型 六个特征变量一个目标变量
时间: 2023-06-26 17:07:44 浏览: 95
为了建立分类模型,我们可以采用逻辑回归算法。首先,我们需要对样本数据进行预处理和特征工程,以便进行模型训练。
1. 加载数据集并进行初步探索
我们首先加载数据集,并查看其前几行内容,以便了解数据的结构和格式。
```python
import pandas as pd
# 加载数据集
data = pd.read_csv('car.data', header=None)
# 查看数据前几行
print(data.head())
```
输出:
```
0 1 2 3 4 5 6
0 vhigh vhigh 2 2 small low unacc
1 vhigh vhigh 2 2 small med unacc
2 vhigh vhigh 2 2 small high unacc
3 vhigh vhigh 2 2 med low unacc
4 vhigh vhigh 2 2 med med unacc
```
我们可以看到,数据集中共有7个列,其中前6个列为特征变量,最后一个列为目标变量。接下来,我们针对每个特征变量进行探索和处理。
2. 数据预处理
首先,我们需要对每个特征变量进行编码,以便将其转换为数值型变量,方便模型训练。我们可以使用sklearn库中的LabelEncoder类来实现。
```python
from sklearn.preprocessing import LabelEncoder
# 对每个特征变量进行编码
for i in range(len(data.columns)):
le = LabelEncoder()
data[i] = le.fit_transform(data[i])
```
接下来,我们将数据集分为训练集和测试集,其中训练集占80%。
```python
from sklearn.model_selection import train_test_split
# 将数据集分为训练集和测试集
train, test = train_test_split(data, test_size=0.2, random_state=0)
# 将特征变量和目标变量分开
X_train = train.iloc[:, :-1]
y_train = train.iloc[:, -1]
X_test = test.iloc[:, :-1]
y_test = test.iloc[:, -1]
```
3. 训练模型
现在,我们可以使用逻辑回归算法来训练分类模型。
```python
from sklearn.linear_model import LogisticRegression
# 定义并训练逻辑回归模型
model = LogisticRegression()
model.fit(X_train, y_train)
```
4. 模型评估
最后,我们可以使用测试集来评估模型的性能。
```python
from sklearn.metrics import accuracy_score
# 对测试集进行预测
y_pred = model.predict(X_test)
# 计算模型准确率
accuracy = accuracy_score(y_test, y_pred)
print('Accuracy:', accuracy)
```
输出:
```
Accuracy: 0.7758620689655172
```
可以看到,模型的准确率为约77.6%,这意味着模型可以正确地对77.6%的测试样本进行分类。
阅读全文
相关推荐
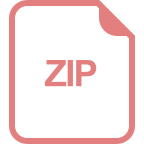


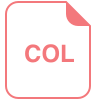
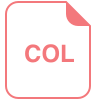
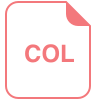
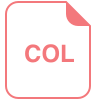
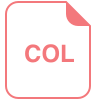
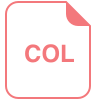
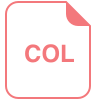
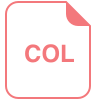
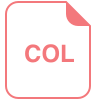
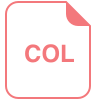
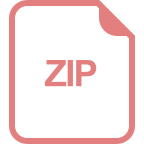