springboot异步调用接口
时间: 2023-07-02 07:04:02 浏览: 139
在Spring Boot中,你可以使用异步调用来提高应用程序的性能和吞吐量。Spring Boot支持两种异步方式:使用Callable和DeferredResult。
使用Callable:
1. 在Controller中定义一个方法,并使用@Async注解标记该方法。
```java
@RestController
public class MyController {
@Autowired
MyService myService;
@GetMapping("/async")
public Callable<String> async() {
return () -> myService.doSomething();
}
}
```
2. 在Service层中定义一个异步方法,并使用@Async注解标记该方法。
```java
@Service
public class MyService {
@Async
public String doSomething() {
// 执行耗时操作
return "result";
}
}
```
使用DeferredResult:
1. 在Controller中定义一个方法,并使用DeferredResult作为返回值。
```java
@RestController
public class MyController {
@Autowired
MyService myService;
@GetMapping("/async")
public DeferredResult<String> async() {
DeferredResult<String> deferredResult = new DeferredResult<>();
CompletableFuture.supplyAsync(() -> myService.doSomething())
.whenCompleteAsync((result, throwable) -> deferredResult.setResult(result));
return deferredResult;
}
}
```
2. 在Service层中定义一个异步方法,并使用CompletableFuture异步执行该方法。
```java
@Service
public class MyService {
public String doSomething() {
// 执行耗时操作
return "result";
}
}
```
注意事项:
1. 需要在Spring Boot应用程序的启动类上添加@EnableAsync注解来启用异步调用。
```java
@SpringBootApplication
@EnableAsync
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
```
2. 异步调用的返回值应该是Future或其子类,例如Callable和DeferredResult。
阅读全文
相关推荐
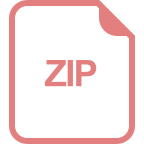
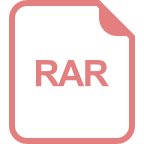
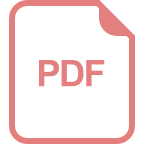
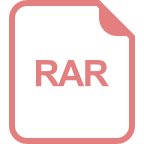
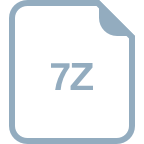

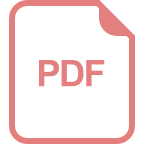
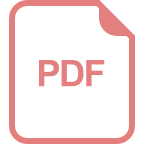
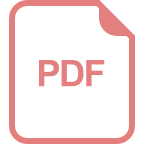
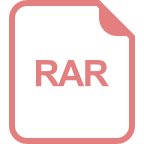
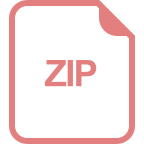
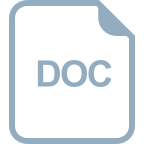





