键盘输入一行字符串,统计空格、数字、中文、英文字符和其他字符个数。基本中文字符的Unicode编码范围是:4E00~9FA5
时间: 2024-05-15 20:16:52 浏览: 35
示例:
输入:Hello, 你好!123
输出:
空格:2
数字:3
中文字符:2
英文字符:9
其他字符:2
代码实现:
```python
s = input() # 输入字符串
space_cnt = 0 # 空格个数
digit_cnt = 0 # 数字个数
chinese_cnt = 0 # 中文字符个数
english_cnt = 0 # 英文字符个数
other_cnt = 0 # 其他字符个数
for c in s:
if c == ' ':
space_cnt += 1
elif c.isdigit():
digit_cnt += 1
elif '一' <= c <= '\u9fff':
chinese_cnt += 1
elif c.isalpha():
english_cnt += 1
else:
other_cnt += 1
print("空格:%d" % space_cnt)
print("数字:%d" % digit_cnt)
print("中文字符:%d" % chinese_cnt)
print("英文字符:%d" % english_cnt)
print("其他字符:%d" % other_cnt)
```
相关问题
输入字符串,输出字符串的每个字符对应的Unicode码。 输入格式: 输入一行字符串。 输出格式: 输出字符串的每个字符对应的Unicode码,用空格间隔。
好的,可以使用以下Python代码实现:
```python
string = input("请输入字符串:")
for char in string:
print(ord(char), end=" ")
```
输入一个字符串后,代码会遍历字符串中的每一个字符,使用内置函数`ord()`将字符转换为对应的Unicode码并输出,每个Unicode码之间用空格间隔。注意,`end=" "`是为了让输出的Unicode码不换行。
用Python统计不同字符的个数,用户输入一行字符,统计并输出其中文字符、数字、空格、汉字和其他字符的个数
好的,可以用Python来实现这个功能。以下是代码实现:
```python
# 获取用户输入的字符串
s = input("请输入一行字符:")
# 初始化各种字符的个数
chinese = 0
digit = 0
space = 0
hanzi = 0
others = 0
# 遍历字符串中的每一个字符
for c in s:
if c.isalpha() and '\u4e00' <= c <= '\u9fa5':
# 中文字符
chinese += 1
hanzi += 1
elif c.isdigit():
# 数字
digit += 1
elif c.isspace():
# 空格
space += 1
elif '\u4e00' <= c <= '\u9fa5':
# 汉字
hanzi += 1
else:
# 其他字符
others += 1
# 输出各种字符的个数
print("中文字符:", chinese)
print("数字:", digit)
print("空格:", space)
print("汉字:", hanzi)
print("其他字符:", others)
```
代码中,我们先获取用户输入的字符串,然后遍历字符串中的每一个字符,根据字符的类型把计数器加1,最后输出各种字符的个数。
注意,这里使用了Unicode编码中中文字符的Unicode范围`\u4e00`到`\u9fa5`来判断中文字符和汉字。
阅读全文
相关推荐
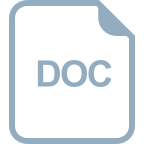













